C++ if, if...else 和嵌套 if...else
C++ if, if...else 和嵌套 if...else
在本教程中,我们将通过示例了解 if...else 语句来创建决策程序。
在计算机编程中,我们使用 if...else
语句在特定条件下运行一段代码,在不同条件下运行另一段代码。
例如,根据学生获得的分数分配等级(A、B、C)。
- 如果百分比高于 90 , 分配等级 A
- 如果百分比高于 75 , 分配等级 B
- 如果百分比高于 65 , 分配等级 C
if...else
的三种形式 C++ 中的语句。
if
声明if...else
声明if...else if...else
声明
C++ if 语句
if
的语法 声明是:
if (condition) {
// body of if statement
}
if
语句评估 condition
括号内 ( )
.
- 如果
condition
计算结果为true
,if
体内的代码 被执行。 - 如果
condition
计算结果为false
,if
体内的代码 被跳过。
注意: { }
里面的代码 是 if
的主体 声明。
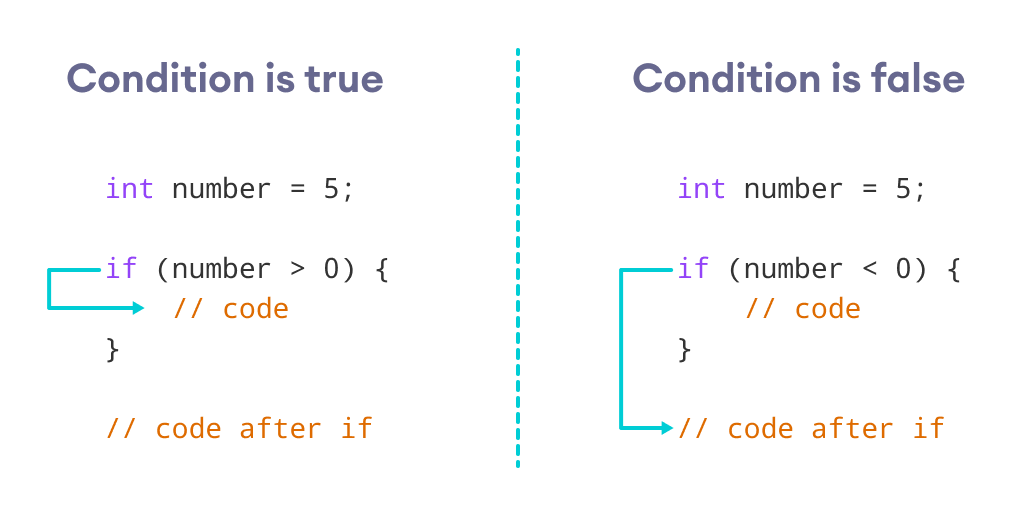
示例1:C++ if 语句
// Program to print positive number entered by the user
// If the user enters a negative number, it is skipped
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
// checks if the number is positive
if (number > 0) {
cout << "You entered a positive integer: " << number << endl;
}
cout << "This statement is always executed.";
return 0;
}
输出 1
Enter an integer: 5 You entered a positive number: 5 This statement is always executed.
当用户输入5
, 条件 number > 0
被评估为 true
以及 if
正文中的语句 被执行。
输出 2
Enter a number: -5 This statement is always executed.
当用户输入-5
, 条件 number > 0
被评估为 false
以及 if
正文中的语句 没有被执行。
C++ if...else
if
语句可以有一个可选的 else
条款。它的语法是:
if (condition) {
// block of code if condition is true
}
else {
// block of code if condition is false
}
if..else
语句评估 condition
括号内。
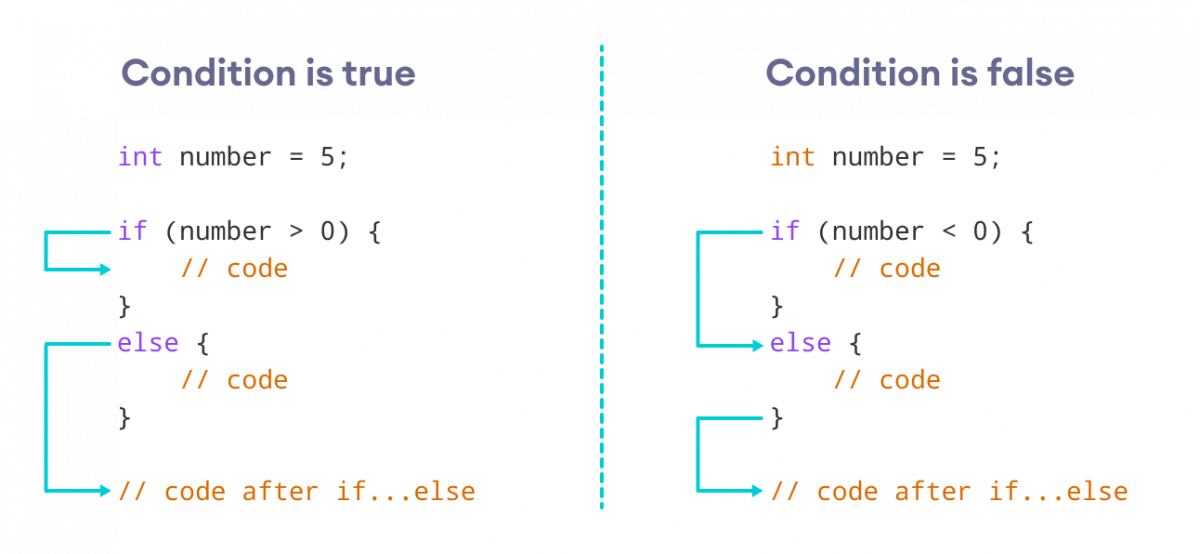
如果 condition
评估 true
,
if
主体内的代码 被执行else
主体内的代码 被跳过执行
如果 condition
评估 false
,
else
主体内的代码 被执行if
主体内的代码 被跳过执行
示例 2:C++ if...else 语句
// Program to check whether an integer is positive or negative
// This program considers 0 as a positive number
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
if (number >= 0) {
cout << "You entered a positive integer: " << number << endl;
}
else {
cout << "You entered a negative integer: " << number << endl;
}
cout << "This line is always printed.";
return 0;
}
输出 1
Enter an integer: 4 You entered a positive integer: 4. This line is always printed.
在上面的程序中,我们有条件 number >= 0
.如果我们输入大于或等于 0 的数字 ,然后条件评估 true
.
在这里,我们输入 4 .所以,条件是 true
.因此,if
主体内的语句 被执行。
输出 2
Enter an integer: -4 You entered a negative integer: -4. This line is always printed.
在这里,我们输入 -4 .所以,条件是 false
.因此,else
主体内的语句 被执行。
C++ if...else...else if 语句
if...else
语句用于在两个备选方案中执行代码块。但是,如果我们需要在两个以上的选择之间做出选择,我们使用 if...else if...else
声明。
if...else if...else
的语法 声明是:
if (condition1) {
// code block 1
}
else if (condition2){
// code block 2
}
else {
// code block 3
}
在这里,
- 如果
condition1
计算结果为true
,code block 1
被执行。 - 如果
condition1
计算结果为false
,然后是condition2
被评估。 - 如果
condition2
是true
,code block 2
被执行。 - 如果
condition2
是false
,code block 3
被执行。
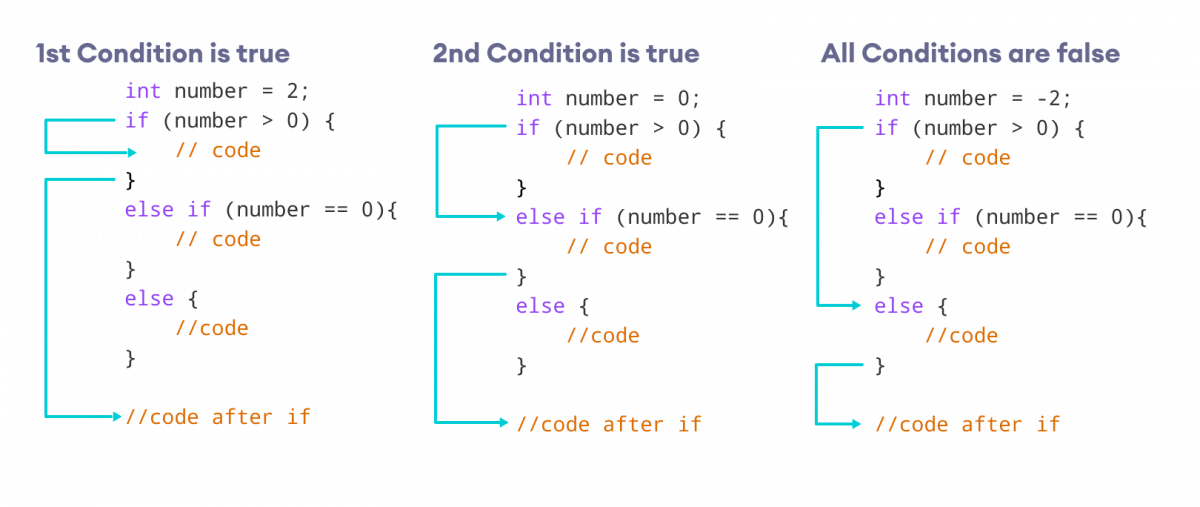
注意: else if
可以有多个 声明但只有一个 if
和 else
声明。
示例 3:C++ if...else...else if
// Program to check whether an integer is positive, negative or zero
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter an integer: ";
cin >> number;
if (number > 0) {
cout << "You entered a positive integer: " << number << endl;
}
else if (number < 0) {
cout << "You entered a negative integer: " << number << endl;
}
else {
cout << "You entered 0." << endl;
}
cout << "This line is always printed.";
return 0;
}
输出 1
Enter an integer: 1 You entered a positive integer: 1. This line is always printed.
输出 2
Enter an integer: -2 You entered a negative integer: -2. This line is always printed.
输出 3
Enter an integer: 0 You entered 0. This line is always printed.
在这个程序中,我们从用户那里获取一个数字。然后我们使用 if...else if...else
梯子来检查数字是正数、负数还是零。
如果数字大于0
,if
里面的代码 块被执行。如果数字小于0
,else if
里面的代码 块被执行。否则,else
里面的代码 块被执行。
C++ 嵌套 if...else
有时,我们需要使用 if
另一个 if
中的语句 陈述。这称为嵌套 if
声明。
把它想象成多层 if
陈述。有一个第一个,外部 if
声明,里面是另一个,内部的 if
陈述。它的语法是:
// outer if statement
if (condition1) {
// statements
// inner if statement
if (condition2) {
// statements
}
}
注意事项:
- 我们可以添加
else
和else if
对内部if
的语句 根据需要声明。 - 内层
if
语句也可以插入到外部else
内 或else if
语句(如果存在)。 - 我们可以嵌套多层
if
声明。
示例 4:C++ 嵌套 if
// C++ program to find if an integer is positive, negative or zero
// using nested if statements
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter an integer: ";
cin >> num;
// outer if condition
if (num != 0) {
// inner if condition
if (num > 0) {
cout << "The number is positive." << endl;
}
// inner else condition
else {
cout << "The number is negative." << endl;
}
}
// outer else condition
else {
cout << "The number is 0 and it is neither positive nor negative." << endl;
}
cout << "This line is always printed." << endl;
return 0;
}
输出 1
Enter an integer: 35 The number is positive. This line is always printed.
输出 2
Enter an integer: -35 The number is negative. This line is always printed.
输出 3
Enter an integer: 0 The number is 0 and it is neither positive nor negative. This line is always printed.
在上面的例子中,
- 我们将一个整数作为用户的输入并将其存储在变量num中 .
- 然后我们使用
if...else
检查 num 是否不等于0
的语句 .- 如果
true
,然后是内部if...else
语句被执行。 - 如果
false
, outer里面的代码else
条件被执行,打印出"The number is 0 and it is neither positive nor negative."
- 如果
- 内在
if...else
语句检查输入数字是否为正,即如果 num 大于 0 .- 如果
true
,然后我们打印一个声明,说这个数字是正数。 - 如果
false
,我们打印出这个数字是负数。
- 如果
注意: 如您所见,嵌套的 if...else
让你的逻辑变得复杂。如果可能,您应该始终尽量避免嵌套 if...else
.
if...else 的主体,只有一个语句
如果 if...else
的正文 只有一个语句,你可以省略 { }
在节目中。比如你可以替换
int number = 5;
if (number > 0) {
cout << "The number is positive." << endl;
}
else {
cout << "The number is negative." << endl;
}
与
int number = 5;
if (number > 0)
cout << "The number is positive." << endl;
else
cout << "The number is negative." << endl;
两个程序的输出是一样的。
注意: 虽然没有必要使用 { }
如果 if...else
的主体 只有一个语句,使用 { }
使您的代码更具可读性。
更多决策
在某些情况下,三元运算符 可以替换 if...else
陈述。要了解更多信息,请访问 C++ 三元运算符。
如果我们需要根据给定的测试条件在多个备选方案之间做出选择,switch
可以使用语句。要了解更多信息,请访问 C++ 开关。
查看这些示例以了解更多信息:
判断数字是偶数还是奇数的C++程序
C++程序检查一个字符是元音还是辅音。
求三个数中最大数的C++程序
C语言