C++ 继承
C++ 继承
在本教程中,我们将通过示例了解 C++ 中的继承。
继承是 C++ 中面向对象编程的关键特性之一。它允许我们从现有的类(基类)创建一个新的类(派生类)。
派生类继承基类的特性 并且可以有自己的附加功能。例如,
class Animal {
// eat() function
// sleep() function
};
class Dog : public Animal {
// bark() function
};
这里,Dog
类派生自 Animal
班级。从 Dog
派生自 Animal
, Animal
的成员 Dog
可以访问 .
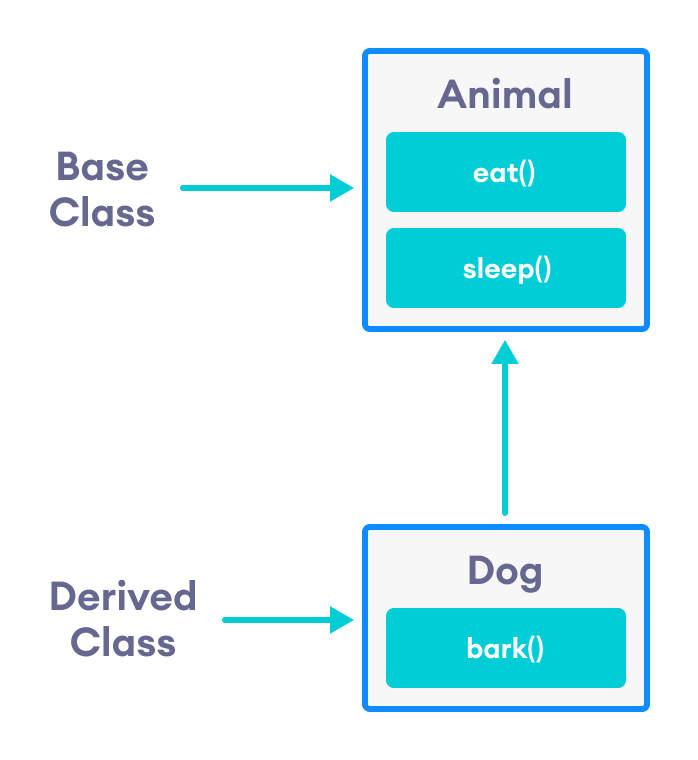
注意关键字 public
的使用 同时从 Animal 继承 Dog。
class Dog : public Animal {...};
我们也可以使用关键字private
和 protected
而不是 public
.我们将了解使用 private
之间的区别 , public
和 protected
本教程的后面部分。
is-a 关系
继承是一种是一种关系 .我们仅在 is-a 关系 时使用继承 存在于两个类之间。
以下是一些例子:
- 汽车就是交通工具。
- 橙子是一种水果。
- 外科医生就是医生。
- 狗是动物。
示例1:C++继承的简单示例
// C++ program to demonstrate inheritance
#include <iostream>
using namespace std;
// base class
class Animal {
public:
void eat() {
cout << "I can eat!" << endl;
}
void sleep() {
cout << "I can sleep!" << endl;
}
};
// derived class
class Dog : public Animal {
public:
void bark() {
cout << "I can bark! Woof woof!!" << endl;
}
};
int main() {
// Create object of the Dog class
Dog dog1;
// Calling members of the base class
dog1.eat();
dog1.sleep();
// Calling member of the derived class
dog1.bark();
return 0;
}
输出
I can eat! I can sleep! I can bark! Woof woof!!
在这里,dog1 (派生类的对象Dog
) 可以访问基类 Animal
的成员 .这是因为 Dog
继承自 Animal
.
// Calling members of the Animal class
dog1.eat();
dog1.sleep();
C++ 保护成员
访问修饰符 protected
在涉及 C++ 继承时尤其重要。
喜欢 private
成员,protected
成员在类之外是不可访问的。但是,它们可以通过派生类访问 和朋友类/功能 .
我们需要 protected
成员,如果我们想隐藏一个类的数据,但仍然希望该数据被其派生类继承。
要了解有关受保护的更多信息,请参阅我们的 C++ 访问修饰符教程。
示例 2:C++ 保护成员
// C++ program to demonstrate protected members
#include <iostream>
#include <string>
using namespace std;
// base class
class Animal {
private:
string color;
protected:
string type;
public:
void eat() {
cout << "I can eat!" << endl;
}
void sleep() {
cout << "I can sleep!" << endl;
}
void setColor(string clr) {
color = clr;
}
string getColor() {
return color;
}
};
// derived class
class Dog : public Animal {
public:
void setType(string tp) {
type = tp;
}
void displayInfo(string c) {
cout << "I am a " << type << endl;
cout << "My color is " << c << endl;
}
void bark() {
cout << "I can bark! Woof woof!!" << endl;
}
};
int main() {
// Create object of the Dog class
Dog dog1;
// Calling members of the base class
dog1.eat();
dog1.sleep();
dog1.setColor("black");
// Calling member of the derived class
dog1.bark();
dog1.setType("mammal");
// Using getColor() of dog1 as argument
// getColor() returns string data
dog1.displayInfo(dog1.getColor());
return 0;
}
输出
I can eat! I can sleep! I can bark! Woof woof!! I am a mammal My color is black
这里,变量 type 是 protected
因此可以从派生类 Dog
访问 .我们可以看到这一点,因为我们已经初始化了 type
在 Dog
使用函数 setType()
的类 .
另一方面,private
变量颜色 无法在 Dog
中初始化 .
class Dog : public Animal {
public:
void setColor(string clr) {
// Error: member "Animal::color" is inaccessible
color = clr;
}
};
另外,由于 protected
关键字隐藏数据,我们无法访问 type 直接来自 Dog
的对象 或 Animal
类。
// Error: member "Animal::type" is inaccessible
dog1.type = "mammal";
C++ 继承中的访问模式
在我们之前的教程中,我们了解了 C++ 访问说明符,例如 public、private 和 protected。
到目前为止,我们已经使用了 public
关键字,以便从先前存在的基类继承类。但是,我们也可以使用 private
和 protected
继承类的关键字。例如,
class Animal {
// code
};
class Dog : private Animal {
// code
};
class Cat : protected Animal {
// code
};
我们可以派生类的各种方式称为访问模式 .这些访问模式的作用如下:
- 公开: 如果在
public
中声明了派生类 模式,那么基类的成员就照原样被派生类继承。 - 私人: 在这种情况下,基类的所有成员都变为
private
派生类中的成员。 - 受保护:
public
基类的成员变为protected
派生类中的成员。
private
基类的成员总是 private
在派生类中。
要了解更多信息,请访问我们的 C++ 公有、私有、受保护的继承教程。
继承中的成员函数重写
假设基类和派生类有同名同参数的成员函数。
如果我们创建派生类的对象并尝试访问该成员函数,则会调用派生类中的成员函数,而不是基类中的成员函数。
派生类的成员函数覆盖基类的成员函数。
详细了解 C++ 中的函数覆盖。
推荐阅读: C++ 多重继承
C语言