C# 数组
C# 数组
在本教程中,我们将学习 C# 数组。我们将通过示例学习创建、初始化和访问数组。
数组是相似类型数据的集合。例如,
假设我们需要记录 5 个学生的年龄。我们可以简单地创建一个数组,而不是创建 5 个单独的变量:
<图>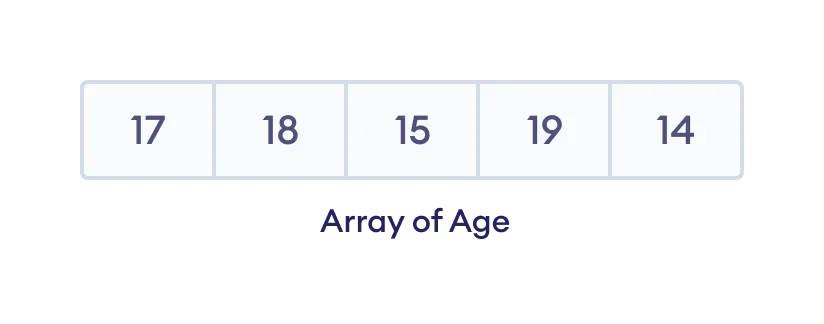
1. C# 数组声明
在 C# 中,我们可以这样声明一个数组。
datatype[] arrayName;
在这里,
dataType
- 像int
这样的数据类型 ,string
,char
等arrayName
- 它是一个标识符
我们来看一个例子,
int[] age;
在这里,我们创建了一个名为 age 的数组 .可以存储int
的元素 输入。
但是它可以存储多少元素呢?
要定义数组可以容纳的元素数量,我们必须在 C# 中为数组分配内存。例如,
// declare an array
int[] age;
// allocate memory for array
age = new int[5];
这里,new int[5]
表示数组可以存储5个元素。我们也可以说数组的大小/长度是5。
注意 :我们也可以在一行中声明和分配数组的内存。例如,
int[] age = new int[5];
2. C#中的数组初始化
在 C# 中,我们可以在声明期间初始化一个数组。例如,
int [] numbers = {1, 2, 3, 4, 5};
在这里,我们创建了一个名为 numbers 的数组并使用值 1 对其进行初始化 , 2 , 3 , 4 , 和 5 在花括号内。
请注意,我们没有提供数组的大小。在这种情况下,C# 会通过计算数组中元素的数量(即 5)来自动指定大小。
在数组中,我们使用索引号 确定每个数组元素的位置。我们可以使用索引号在 C# 中初始化一个数组。例如,
// declare an array
int[] age = new int[5];
//initializing array
age[0] = 12;
age[1] = 4;
age[2] = 5;
...
<图> 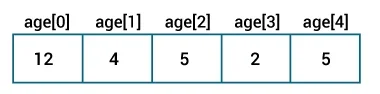
注意 :
- 数组索引始终从 0 开始。也就是说,数组的第一个元素位于索引 0。
- 如果数组的大小为 5,则最后一个元素的索引将为 4 (5 - 1)。
3.访问数组元素
我们可以使用数组的索引来访问数组中的元素。例如,
// access element at index 2
array[2];
// access element at index 4
array[4];
在这里,
array[2]
- 访问第三个元素array[4]
- 访问第 5 个元素
示例:C# 数组
using System;
namespace AccessArray {
class Program {
static void Main(string[] args) {
// create an array
int[] numbers = {1, 2, 3};
//access first element
Console.WriteLine("Element in first index : " + numbers[0]);
//access second element
Console.WriteLine("Element in second index : " + numbers[1]);
//access third element
Console.WriteLine("Element in third index : " + numbers[2]);
Console.ReadLine();
}
}
}
输出
Element in first index : 1 Element in second index : 2 Element in third index : 3
在上面的示例中,我们创建了一个名为 numbers 的数组 带有元素 1、2、3 .在这里,我们使用索引号 访问数组的元素。
numbers[0]
- 访问第一个元素,1numbers[1]
- 访问第二个元素,2numbers[3]
- 访问第三个元素,3
4.更改数组元素
我们还可以更改数组的元素。要更改元素,我们只需为该特定索引分配一个新值。例如,
using System;
namespace ChangeArray {
class Program {
static void Main(string[] args) {
// create an array
int[] numbers = {1, 2, 3};
Console.WriteLine("Old Value at index 0: " + numbers[0]);
// change the value at index 0
numbers[0] = 11;
//print new value
Console.WriteLine("New Value at index 0: " + numbers[0]);
Console.ReadLine();
}
}
}
输出
Old Value at index 0: 1 New Value at index 0: 11
在上面的例子中,索引 0 处的初始值为 1。注意这一行,
//change the value at index 0
numbers[0] = 11;
在这里,我们分配了一个新值 11 到索引 0。现在,索引 0 处的值从 1 更改 到11 .
5。使用循环迭代 C# 数组
在 C# 中,我们可以使用循环来遍历数组的每个元素。例如,
示例:使用 for 循环
using System;
namespace AccessArrayFor {
class Program {
static void Main(string[] args) {
int[] numbers = { 1, 2, 3};
for(int i=0; i < numbers.Length; i++) {
Console.WriteLine("Element in index " + i + ": " + numbers[i]);
}
Console.ReadLine();
}
}
}
输出
Element in index 0: 1 Element in index 1: 2 Element in index 2: 3
在上面的例子中,我们使用了一个 for 循环来遍历数组的元素,numbers .注意这一行,
numbers.Length
这里,Length
数组的属性给出了数组的大小。
我们还可以使用 foreach 循环来遍历数组的元素。例如,
示例:使用 foreach 循环
using System;
namespace AccessArrayForeach {
class Program {
static void Main(string[] args) {
int[] numbers = {1, 2, 3};
Console.WriteLine("Array Elements: ");
foreach(int num in numbers) {
Console.WriteLine(num);
}
Console.ReadLine();
}
}
}
输出
Array Elements: 1 2 3
6.使用 System.Linq 的 C# 数组操作
在 C# 中,我们有 System.Linq
命名空间,提供不同的方法来执行数组中的各种操作。例如,
示例:查找最小和最大元素
using System;
// provides us various methods to use in an array
using System.Linq;
namespace ArrayMinMax {
class Program {
static void Main(string[] args) {
int[] numbers = {51, 1, 3, 4, 98};
// get the minimum element
Console.WriteLine("Smallest Element: " + numbers.Min());
// Max() returns the largest number in array
Console.WriteLine("Largest Element: " + numbers.Max());
Console.ReadLine();
}
}
}
输出
Smallest Element: 1 Largest Element: 98
在上面的例子中,
numbers.Min()
- 返回数组中的最小数,1numbers.Max()
- 返回数组中的最大数,98
示例:求数组的平均值
using System;
// provides us various methods to use in an array
using System.Linq;
namespace ArrayFunction {
class Program {
static void Main(string[] args) {
int[] numbers = {30, 31, 94, 86, 55};
// get the sum of all array elements
float sum = numbers.Sum();
// get the total number of elements present in the array
int count = numbers.Count();
float average = sum/count;
Console.WriteLine("Average : " + average);
// compute the average
Console.WriteLine("Average using Average() : " + numbers.Average());
Console.ReadLine();
}
}
}
输出
Average : 59.2 Average using Average() : 59.2
在上面的例子中,我们使用了
numbers.Sum()
得到数组所有元素的总和numbers.Count()
获取数组中存在的元素总数
然后我们将总和除以计数得到平均值。
float average = sum / count;
在这里,我们也使用了 numbers.Average()
System.Linq
的方法 namespace 直接取平均值。
注意 :必须使用 System.Linq
使用 Min()
时的命名空间 , Max()
, Sum()
, Count()
, 和 Average()
方法。
C语言