C# continue 语句
C# continue 语句
在本教程中,您将通过示例了解 C# continue 语句的工作原理。
在 C# 中,我们使用 continue 语句跳过循环的当前迭代。
当我们的程序遇到 continue 语句时,程序控制移动到循环的末尾并执行测试条件(for 循环时的更新语句)。
continue 的语法是:
continue;
在我们了解继续之前,请务必了解
- for循环
- while 循环
- 如果...否则
Example1:C# continue with for loop
using System;
namespace ContinueLoop {
class Program {
static void Main(string[] args){
for (int i = 1; i <= 5; ++i{
if (i == 3) {
continue;
}
Console.WriteLine(i);
}
}
}
}
输出
1 2 4 5
在上面的例子中,我们使用了 for 循环来打印 i =1 到 5 的数字 .然而,数字 3 没有打印出来。
这是因为当 i 的值 是 3 , continue
语句被执行。
// skips the condition
if (i == 3) {
continue;
}
这将跳过循环的当前迭代并将程序控制移至更新语句。因此,值 3 没有打印出来。
注意 :continue 语句通常与 if...else 语句一起使用。
示例:C# 使用 while 循环继续
using System;
namespace ContinueWhile {
class Program{
static void Main(string[] args) {
int i = 0;
while (i < 5) {
i++;
if (i == 3) {
continue;
}
Console.WriteLine(i);
}
}
}
}
输出
1 2 4 5
在这里,我们使用了 continue
while
内的语句 环形。与前面的程序类似,当 i 的值 是 3 ,继续执行语句。
因此,3 不打印在屏幕上。
C# continue 语句的工作
<图>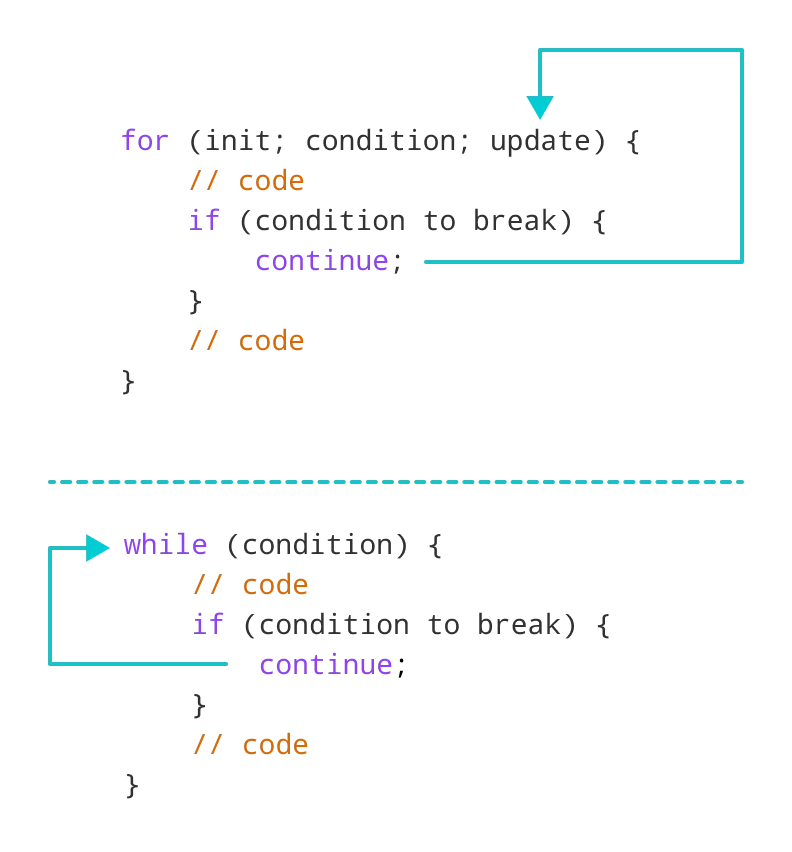
继续嵌套循环
我们也使用带有嵌套的 continue 语句。例如:
using System;
namespace ContinueNested {
class Program {
static void Main(string[] args) {
int sum = 0;
// outer loop
for(int i = 1; i <= 3; i++) {
// inner loop
for(int j = 1; j <= 3; j++) {
if (j == 2) {
continue;
}
Console.WriteLine("i = " + i + " j = " +j);
}
}
}
}
}
输出
i = 1 j = 1 i = 1 j = 3 i = 2 j = 1 i = 2 j = 3 i = 3 j = 1 i = 3 j = 3
在上面的例子中,我们在 for
内部使用了 continue 语句 环形。这里, continue 语句在 j == 2
时执行 .
因此,j = 2
的值 被忽略。
如果您想了解嵌套循环的工作原理,请访问 C# Nested Loops。
C# 继续 foreach 循环
我们也可以使用 continue
带有 foreach 循环的语句。例如,
using System;
namespace ContinueForeach {
class Program {
static void Main(string[] args) {
int[] num = { 1, 2, 3, 4, 5 };
foreach(int number in num) {
// skips the iteration
if(number==3) {
continue;
}
Console.WriteLine(number);
}
}
}
}
输出
1 2 4 5
在上面的示例中,我们创建了一个包含值的数组:1 , 2 , 3 , 4 , 5 .在这里,我们使用了 foreach 循环来打印数组的每个元素。
但是,循环不会打印值 3 .这是因为当数字等于 3 , continue
语句被执行。
if (number == 3) {
continue;
}
因此,跳过了本次迭代的打印语句。
C语言