Python 集
Python 集
在本教程中,您将了解有关 Python 集的所有内容;它们是如何被创建、添加或删除元素的,以及在 Python 中对集合执行的所有操作。
视频:Python 中的集合
集合是项目的无序集合。每个集合元素都是唯一的(没有重复)并且必须是不可变的(不能更改)。
但是,集合本身是可变的。我们可以从中添加或删除项目。
集合还可以用于执行数学集合运算,例如并集、交集、对称差等。
创建 Python 集
通过将所有项目(元素)放在花括号 {}
内来创建一个集合 , 用逗号分隔,或者使用内置的 set()
功能。
它可以有任意数量的项目,它们可以是不同的类型(整数、浮点数、元组、字符串等)。但是集合不能以列表、集合或字典等可变元素作为其元素。
# Different types of sets in Python
# set of integers
my_set = {1, 2, 3}
print(my_set)
# set of mixed datatypes
my_set = {1.0, "Hello", (1, 2, 3)}
print(my_set)
输出
{1, 2, 3} {1.0, (1, 2, 3), 'Hello'}
也试试下面的例子。
# set cannot have duplicates
# Output: {1, 2, 3, 4}
my_set = {1, 2, 3, 4, 3, 2}
print(my_set)
# we can make set from a list
# Output: {1, 2, 3}
my_set = set([1, 2, 3, 2])
print(my_set)
# set cannot have mutable items
# here [3, 4] is a mutable list
# this will cause an error.
my_set = {1, 2, [3, 4]}
输出
{1, 2, 3, 4} {1, 2, 3} Traceback (most recent call last): File "<string>", line 15, in <module> my_set = {1, 2, [3, 4]} TypeError: unhashable type: 'list'
创建一个空集有点棘手。
空花括号 {}
将在 Python 中创建一个空字典。要制作一个没有任何元素的集合,我们使用 set()
没有任何参数的函数。
# Distinguish set and dictionary while creating empty set
# initialize a with {}
a = {}
# check data type of a
print(type(a))
# initialize a with set()
a = set()
# check data type of a
print(type(a))
输出
<class 'dict'> <class 'set'>
在 Python 中修改一个集合
集合是可变的。但是,由于它们是无序的,因此索引没有任何意义。
我们无法使用索引或切片访问或更改集合中的元素。设置数据类型不支持。
我们可以使用 add()
添加单个元素 方法,以及使用 update()
的多个元素 方法。 update()
方法可以将元组、列表、字符串或其他集合作为其参数。在所有情况下,都避免重复。
# initialize my_set
my_set = {1, 3}
print(my_set)
# my_set[0]
# if you uncomment the above line
# you will get an error
# TypeError: 'set' object does not support indexing
# add an element
# Output: {1, 2, 3}
my_set.add(2)
print(my_set)
# add multiple elements
# Output: {1, 2, 3, 4}
my_set.update([2, 3, 4])
print(my_set)
# add list and set
# Output: {1, 2, 3, 4, 5, 6, 8}
my_set.update([4, 5], {1, 6, 8})
print(my_set)
输出
{1, 3} {1, 2, 3} {1, 2, 3, 4} {1, 2, 3, 4, 5, 6, 8}
从集合中移除元素
可以使用 discard()
方法从集合中删除特定项目 和 remove()
.
两者的唯一区别是 discard()
如果元素不存在于集合中,则函数保持集合不变。另一方面,remove()
函数将在这种情况下引发错误(如果集合中不存在元素)。
下面的例子将说明这一点。
# Difference between discard() and remove()
# initialize my_set
my_set = {1, 3, 4, 5, 6}
print(my_set)
# discard an element
# Output: {1, 3, 5, 6}
my_set.discard(4)
print(my_set)
# remove an element
# Output: {1, 3, 5}
my_set.remove(6)
print(my_set)
# discard an element
# not present in my_set
# Output: {1, 3, 5}
my_set.discard(2)
print(my_set)
# remove an element
# not present in my_set
# you will get an error.
# Output: KeyError
my_set.remove(2)
输出
{1, 3, 4, 5, 6} {1, 3, 5, 6} {1, 3, 5} {1, 3, 5} Traceback (most recent call last): File "<string>", line 28, in <module> KeyError: 2
同样,我们可以使用 pop()
移除并返回一个项目 方法。
由于 set 是一种无序数据类型,因此无法确定将弹出哪个项目。这是完全任意的。
我们还可以使用 clear()
从集合中删除所有项目 方法。
# initialize my_set
# Output: set of unique elements
my_set = set("HelloWorld")
print(my_set)
# pop an element
# Output: random element
print(my_set.pop())
# pop another element
my_set.pop()
print(my_set)
# clear my_set
# Output: set()
my_set.clear()
print(my_set)
print(my_set)
输出
{'H', 'l', 'r', 'W', 'o', 'd', 'e'} H {'r', 'W', 'o', 'd', 'e'} set()
Python 集合操作
集合可用于执行数学集合运算,如并集、交集、差分和对称差分。我们可以使用运算符或方法来做到这一点。
让我们考虑以下两组的操作。
>>> A = {1, 2, 3, 4, 5}
>>> B = {4, 5, 6, 7, 8}
设置联合
<图>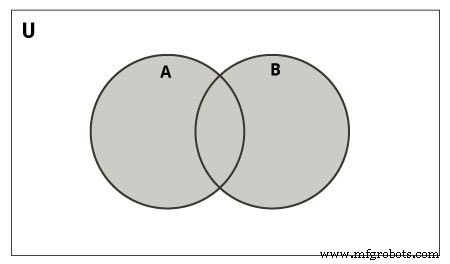
A 的联合 和 B 是两个集合中所有元素的集合。
使用 |
执行联合 操作员。同样可以使用 union()
来完成 方法。
# Set union method
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use | operator
# Output: {1, 2, 3, 4, 5, 6, 7, 8}
print(A | B)
输出
{1, 2, 3, 4, 5, 6, 7, 8}
在 Python shell 上尝试以下示例。
# use union function
>>> A.union(B)
{1, 2, 3, 4, 5, 6, 7, 8}
# use union function on B
>>> B.union(A)
{1, 2, 3, 4, 5, 6, 7, 8}
设置交点
<图>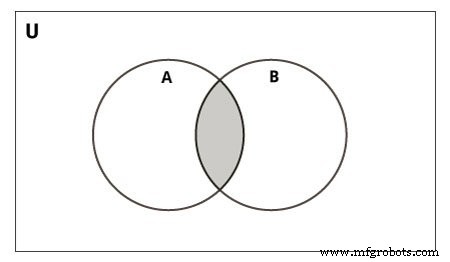
A 的交点 和 B 是两个集合中共有的一组元素。
使用 &
执行交叉 操作员。同样可以使用 intersection()
来完成 方法。
# Intersection of sets
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use & operator
# Output: {4, 5}
print(A & B)
输出
{4, 5}
在 Python shell 上尝试以下示例。
# use intersection function on A
>>> A.intersection(B)
{4, 5}
# use intersection function on B
>>> B.intersection(A)
{4, 5}
设置差异
<图>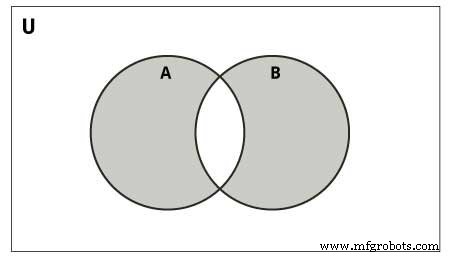
集合 B 的差异 从集合 A (A - B ) 是一组仅在 A 中的元素 但不在 B 中 .同样,B - A 是 B 中的一组元素 但不在 A 中 .
使用 -
执行差异 操作员。同样可以使用 difference()
来完成 方法。
# Difference of two sets
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use - operator on A
# Output: {1, 2, 3}
print(A - B)
输出
{1, 2, 3}
在 Python shell 上尝试以下示例。
# use difference function on A
>>> A.difference(B)
{1, 2, 3}
# use - operator on B
>>> B - A
{8, 6, 7}
# use difference function on B
>>> B.difference(A)
{8, 6, 7}
设置对称差
<图>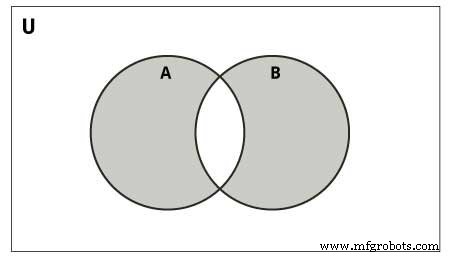
A 的对称差 和 B 是 A 中的一组元素 和 B 但不在两者中(不包括交叉点)。
使用 ^
进行对称差分 操作员。同样可以使用 symmetric_difference()
方法完成 .
# Symmetric difference of two sets
# initialize A and B
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
# use ^ operator
# Output: {1, 2, 3, 6, 7, 8}
print(A ^ B)
输出
{1, 2, 3, 6, 7, 8}
在 Python shell 上尝试以下示例。
# use symmetric_difference function on A
>>> A.symmetric_difference(B)
{1, 2, 3, 6, 7, 8}
# use symmetric_difference function on B
>>> B.symmetric_difference(A)
{1, 2, 3, 6, 7, 8}
其他 Python 集合方法
设置方法有很多,其中一些我们在上面已经使用过了。以下是集合对象可用的所有方法的列表:
方法 | 说明 |
---|---|
add() | 向集合中添加一个元素 |
clear() | 从集合中移除所有元素 |
复制() | 返回集合的副本 |
差异() | 将两个或多个集合的差异作为新集合返回 |
difference_update() | 从这个集合中移除另一个集合的所有元素 |
丢弃() | 如果元素是成员,则从集合中删除它。 (如果元素不在集合中,什么也不做) |
intersection() | 将两个集合的交集作为一个新集合返回 |
intersection_update() | 用自己和另一个的交集更新集合 |
isdisjoint() | 返回 True 如果两个集合有一个空交集 |
issubset() | 返回 True 如果另一个集合包含这个集合 |
issuperset() | 返回 True 如果这个集合包含另一个集合 |
pop() | 移除并返回任意集合元素。引发 KeyError 如果集合为空 |
删除() | 从集合中移除一个元素。如果元素不是成员,则引发 KeyError |
对称差分() | 将两个集合的对称差作为新集合返回 |
对称差分更新() | 用自己和另一个的对称差更新一个集合 |
联合() | 返回新集合中的集合并集 |
更新() | 用自己和其他人的并集更新集合 |
其他集合操作
设置会员测试
我们可以使用 in
测试一个项目是否存在于集合中 关键字。
# in keyword in a set
# initialize my_set
my_set = set("apple")
# check if 'a' is present
# Output: True
print('a' in my_set)
# check if 'p' is present
# Output: False
print('p' not in my_set)
输出
True False
遍历集合
我们可以使用 for
遍历集合中的每个项目 循环。
>>> for letter in set("apple"):
... print(letter)
...
a
p
e
l
带集合的内置函数
all()
等内置函数 , any()
, enumerate()
, len()
, max()
, min()
, sorted()
, sum()
等通常与集合一起使用以执行不同的任务。
函数 | 说明 |
---|---|
all() | 返回 True 如果集合的所有元素都为真(或者集合为空)。 |
any() | 返回 True 如果集合中的任何元素为真。如果集合为空,则返回 False . |
枚举() | 返回一个枚举对象。它包含集合中所有项目的索引和值。 |
len() | 返回集合中的长度(项目数)。 |
max() | 返回集合中最大的项目。 |
min() | 返回集合中最小的项。 |
排序() | 从集合中的元素返回一个新的排序列表(不对集合本身进行排序)。 |
sum() | 返回集合中所有元素的总和。 |
Python Frozenset
Frozenset 是一个新的类,具有集合的特性,但它的元素一旦被赋值就不能改变。元组是不可变的列表,而frozensets 是不可变的集合。
可变的集合是不可散列的,因此它们不能用作字典键。另一方面,frozensets 是可散列的,可以用作字典的键。
可以使用 freezeset() 函数创建冻结集。
此数据类型支持 copy()
等方法 , difference()
, intersection()
, isdisjoint()
, issubset()
, issuperset()
, symmetric_difference()
和 union()
.作为不可变的,它没有添加或删除元素的方法。
# Frozensets
# initialize A and B
A = frozenset([1, 2, 3, 4])
B = frozenset([3, 4, 5, 6])
在 Python shell 上尝试这些示例。
>>> A.isdisjoint(B)
False
>>> A.difference(B)
frozenset({1, 2})
>>> A | B
frozenset({1, 2, 3, 4, 5, 6})
>>> A.add(3)
...
AttributeError: 'frozenset' object has no attribute 'add'
Python