C# 继承
C#继承
在本教程中,我们将通过示例了解 C# 继承及其类型。
在 C# 中,继承允许我们从现有类创建一个新类。它是面向对象编程(OOP)的一个关键特性。
创建新类的类称为基类(父类或超类)。并且,新类称为派生类(子类或子类)
派生类继承基类的字段和方法。这有助于 C# 中的代码可重用性。
如何在C#中进行继承?
在 C# 中,我们使用 :
执行继承的符号。例如,
class Animal {
// fields and methods
}
// Dog inherits from Animal
class Dog : Animal {
// fields and methods of Animal
// fields and methods of Dog
}
在这里,我们继承了派生类 Dog 来自基类 Animal . 狗 类现在可以访问 Animal 的字段和方法 类。<图>
示例:C# 继承
using System;
namespace Inheritance {
// base class
class Animal {
public string name;
public void display() {
Console.WriteLine("I am an animal");
}
}
// derived class of Animal
class Dog : Animal {
public void getName() {
Console.WriteLine("My name is " + name);
}
}
class Program {
static void Main(string[] args) {
// object of derived class
Dog labrador = new Dog();
// access field and method of base class
labrador.name = "Rohu";
labrador.display();
// access method from own class
labrador.getName();
Console.ReadLine();
}
}
}
输出
I am an animal My name is Rohu
在上面的例子中,我们派生了一个子类 Dog 来自超类 Animal .注意语句,
labrador.name = "Rohu";
labrador.getName();
在这里,我们使用 labrador (Dog 的对象)访问 name 和 display() 动物 班级。这是可能的,因为派生类继承了基类的所有字段和方法。
此外,我们访问了 name Dog 方法中的字段 类。
is-a 关系
在 C# 中,继承是一种 is-a 关系。仅当两个类之间存在 is-a 关系时,我们才使用继承。例如,
- 狗 是一个动物
- 苹果 是一个水果
- 汽车 是车辆
我们可以推导出 Dog 来自动物 班级。同样,苹果 来自水果 类和汽车 从车辆 类。
C# 继承中受保护的成员
当我们将一个字段或方法声明为 protected
,只能从同一个类及其派生类访问。
示例:继承中的受保护成员
using System;
namespace Inheritance {
// base class
class Animal {
protected void eat() {
Console.WriteLine("I can eat");
}
}
// derived class of Animal
class Dog : Animal {
static void Main(string[] args) {
Dog labrador = new Dog();
// access protected method from base class
labrador.eat();
Console.ReadLine();
}
}
}
输出
I can eat
在上面的例子中,我们创建了一个名为 Animal 的类 .该类包括一个受保护的方法 eat() .
我们导出了 Dog Animal 类 班级。注意声明,
labrador.eat();
自protected
方法可以从派生类访问,我们可以访问 eat() Dog 的方法 类。
继承类型
继承有以下几种:
1。单一继承
在单继承中,单个派生类从单个基类继承。
<图>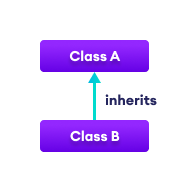
2。多级继承
在多级继承中,派生类从基类继承,然后同一个派生类充当另一个类的基类。
<图>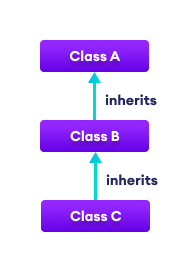
3。分层继承
在层次继承中,多个派生类从一个基类继承。
<图>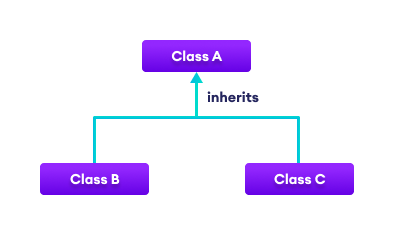
4。多重继承
在多重继承中,一个派生类继承自多个基类。 C# 不支持多重继承。 但是,我们可以通过接口实现多重继承。
<图>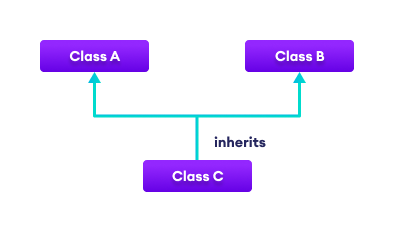
5。混合继承
混合继承是两种或多种继承类型的组合。多级继承和层次继承的结合就是混合继承的一个例子。
<图>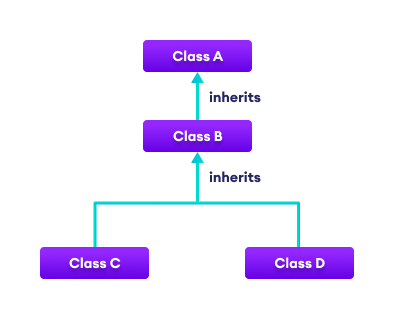
C# 继承中的方法覆盖
如果基类和派生类中都存在相同的方法,则派生类中的方法将覆盖基类中的方法。这在 C# 中称为方法覆盖。例如,
using System;
namespace Inheritance {
// base class
class Animal {
public virtual void eat() {
Console.WriteLine("I eat food");
}
}
// derived class of Animal
class Dog : Animal {
// overriding method from Animal
public override void eat() {
Console.WriteLine("I eat Dog food");
}
}
class Program {
static void Main(string[] args) {
// object of derived class
Dog labrador = new Dog();
// accesses overridden method
labrador.eat();
}
}
}
输出
I eat Dog food
在上面的例子中,eat() 方法存在于基类和派生类中。
当我们调用 eat() 使用 狗 对象 拉布拉多 ,
labrador.eat();
Dog 里面的方法 叫做。这是因为 Dog 里面的方法 覆盖 Animal 中的相同方法 .
注意,我们使用了 virtual
和 覆盖 分别使用基类和派生类的方法。在这里,
virtual
- 允许方法被派生类覆盖override
- 表示该方法正在覆盖基类中的方法
C# 继承中的基本关键字
在前面的例子中,我们看到派生类中的方法覆盖了基类中的方法。
但是,如果我们也想调用基类的方法呢?
在这种情况下,我们使用 base
关键字从派生类调用基类的方法。
示例:C# 继承中的 base 关键字
using System;
namespace Inheritance {
// base class
class Animal {
public virtual void eat() {
Console.WriteLine("Animals eat food.");
}
}
// derived class of Animal
class Dog : Animal {
// overriding method from Animal
public override void eat() {
// call method from Animal class
base.eat();
Console.WriteLine("Dogs eat Dog food.");
}
}
class Program {
static void Main(string[] args) {
Dog labrador = new Dog();
labrador.eat();
}
}
}
输出
Animals eat food. Dogs eat Dog food.
在上面的例子中,eat() 方法存在于两个基类 Animal 和派生类 Dog .注意声明,
base.eat();
在这里,我们使用了 base
访问 Animal 方法的关键字 Dog 的类 类。
C#中继承的重要性
为了理解继承的重要性,让我们考虑一种情况。
假设我们正在使用正多边形,例如正方形、矩形等。而且,我们必须根据输入找到这些多边形的周长。
1. 由于计算周长的公式对于所有正多边形都是通用的,我们可以创建一个 RegularPolygon 类和方法 calculatePerimeter() 计算周长。
class RegularPolygon {
calculatePerimeter() {
// code to compute perimeter
}
}
2. 并继承 Square 和 矩形 RegularPolygon 中的类 班级。这些类中的每一个都将具有存储长度和边数的属性,因为它们对于所有多边形都是不同的。
class Square : RegularPolygon {
int length = 0;
int sides = 0;
}
我们传递 length 的值 和边 calculateperimeter() 计算周长。
这就是继承使我们的代码可重用和更直观的方式。
示例:继承的重要性
using System;
namespace Inheritance {
class RegularPolygon {
public void calculatePerimeter(int length, int sides) {
int result = length * sides;
Console.WriteLine("Perimeter: " + result);
}
}
class Square : RegularPolygon {
public int length = 200;
public int sides = 4;
public void calculateArea() {
int area = length * length;
Console.WriteLine("Area of Square: " + area);
}
}
class Rectangle : RegularPolygon {
public int length = 100;
public int breadth = 200;
public int sides = 4;
public void calculateArea() {
int area = length * breadth;
Console.WriteLine("Area of Rectangle: " + area);
}
}
class Program {
static void Main(string[] args) {
Square s1 = new Square();
s1.calculateArea();
s1.calculatePerimeter(s1.length, s1.sides);
Rectangle t1 = new Rectangle();
t1.calculateArea();
t1.calculatePerimeter(t1.length, t1.sides);
}
}
}
输出
Area of Square: 40000 Perimeter: 800 Area of Rectangle: 20000 Perimeter: 400
在上面的例子中,我们创建了一个 RegularPolygon 具有计算正多边形周长的方法的类。
在这里,Square 和 矩形 继承自 RegularPolygon .
计算周长的公式是通用的,所以我们重用了 calculatePerimeter() 基类的方法。
并且由于不同的形状计算面积的公式不同,所以我们在派生类内部创建了一个单独的方法来计算面积。
C语言