C++ 多级、多级和分层继承
C++ 多重、多级和分层继承
在本教程中,我们将通过示例了解 C++ 编程中不同的继承模型:多继承、多级继承和分层继承。
继承是面向对象编程语言的核心特性之一。它允许软件开发人员从现有类派生新类。派生类继承了基类(现有类)的特性。
C++编程中有多种继承模型。
C++ 多级继承
在 C++ 编程中,不仅可以从基类派生类,还可以从派生类派生类。这种继承形式称为多级继承。
class A { ... .. ... }; class B: public A { ... .. ... }; class C: public B { ... ... ... };
在这里,类 B 派生自基类 A 和类 C 派生自派生类B .
示例 1:C++ 多级继承
#include <iostream>
using namespace std;
class A {
public:
void display() {
cout<<"Base class content.";
}
};
class B : public A {};
class C : public B {};
int main() {
C obj;
obj.display();
return 0;
}
输出
Base class content.
在这个程序中,类 C 派生自类 B (派生自基类 A )。
obj C 类的对象 在main()
中定义 功能。
当display()
函数被调用,display()
在类 A 被执行。这是因为没有display()
C 类中的函数 和类 B .
编译器首先查找 display()
C 类中的函数 .由于该函数在那里不存在,它会在类 B 中查找该函数 (作为 C 派生自 B )。
B 类中也不存在该函数 ,因此编译器在类 A 中查找它 (作为 B 派生自 A )。
如果 display()
C 中存在函数 ,编译器会覆盖 display()
A 类的 (因为成员函数重写)。
C++ 多重继承
在 C++ 编程中,一个类可以从多个父级派生。例如,A 类 Bat 派生自基类 Mammal 和 WingedAnimal .这是有道理的,因为蝙蝠既是哺乳动物又是有翼动物。
<图>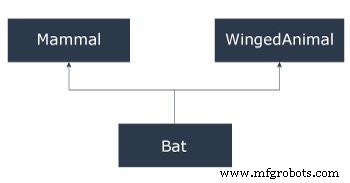
示例 2:C++ 编程中的多重继承
#include <iostream>
using namespace std;
class Mammal {
public:
Mammal() {
cout << "Mammals can give direct birth." << endl;
}
};
class WingedAnimal {
public:
WingedAnimal() {
cout << "Winged animal can flap." << endl;
}
};
class Bat: public Mammal, public WingedAnimal {};
int main() {
Bat b1;
return 0;
}
输出
Mammals can give direct birth. Winged animal can flap.
多重继承中的歧义
多重继承最明显的问题发生在函数覆盖期间。
假设,两个基类有一个相同的函数,在派生类中没有被重写。
如果您尝试使用派生类的对象调用函数,编译器会显示错误。这是因为编译器不知道要调用哪个函数。例如,
class base1 {
public:
void someFunction( ) {....}
};
class base2 {
void someFunction( ) {....}
};
class derived : public base1, public base2 {};
int main() {
derived obj;
obj.someFunction() // Error!
}
可以使用范围解析函数来解决这个问题,以指定将哪个函数归类为 base1 或 base2
int main() { obj.base1::someFunction( ); // Function of base1 class is called obj.base2::someFunction(); // Function of base2 class is called. }
C++ 分层继承
如果从基类继承了多个类,则称为层次继承。在层次继承中,所有子类共有的特性都包含在基类中。
例如,Physics、Chemistry、Biology 都是从 Science 类派生的。同样,Dog、Cat、Horse 都是从 Animal 类派生的。
层次继承的语法
class base_class { ... .. ... } class first_derived_class: public base_class { ... .. ... } class second_derived_class: public base_class { ... .. ... } class third_derived_class: public base_class { ... .. ... }
示例 3:C++ 编程中的层次继承
// C++ program to demonstrate hierarchical inheritance
#include <iostream>
using namespace std;
// base class
class Animal {
public:
void info() {
cout << "I am an animal." << endl;
}
};
// derived class 1
class Dog : public Animal {
public:
void bark() {
cout << "I am a Dog. Woof woof." << endl;
}
};
// derived class 2
class Cat : public Animal {
public:
void meow() {
cout << "I am a Cat. Meow." << endl;
}
};
int main() {
// Create object of Dog class
Dog dog1;
cout << "Dog Class:" << endl;
dog1.info(); // Parent Class function
dog1.bark();
// Create object of Cat class
Cat cat1;
cout << "\nCat Class:" << endl;
cat1.info(); // Parent Class function
cat1.meow();
return 0;
}
输出
Dog Class: I am an animal. I am a Dog. Woof woof. Cat Class: I am an animal. I am a Cat. Meow.
在这里,Dog
和 Cat
类派生自 Animal
班级。因此,两个派生类都可以访问 info()
属于Animal
的函数 类。
C语言