C++ 函数覆盖
C++ 函数覆盖
在本教程中,我们将通过示例了解 C++ 中的函数覆盖。
我们知道,继承是 OOP 的一个特性,它允许我们从基类创建派生类。派生类继承基类的特性。
假设在派生类和基类中都定义了相同的函数。现在如果我们使用派生类的对象调用这个函数,就会执行派生类的函数。
这称为函数覆盖 在 C++ 中。派生类中的函数会覆盖基类中的函数。
示例 1:C++ 函数覆盖
// C++ program to demonstrate function overriding
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1;
derived1.print();
return 0;
}
输出
Derived Function
这里,同样的函数print()
在 Base
中都定义了 和 Derived
类。
所以,当我们调用 print()
来自 Derived
对象 derived1 , print()
来自 Derived
通过覆盖 Base
中的函数来执行 .
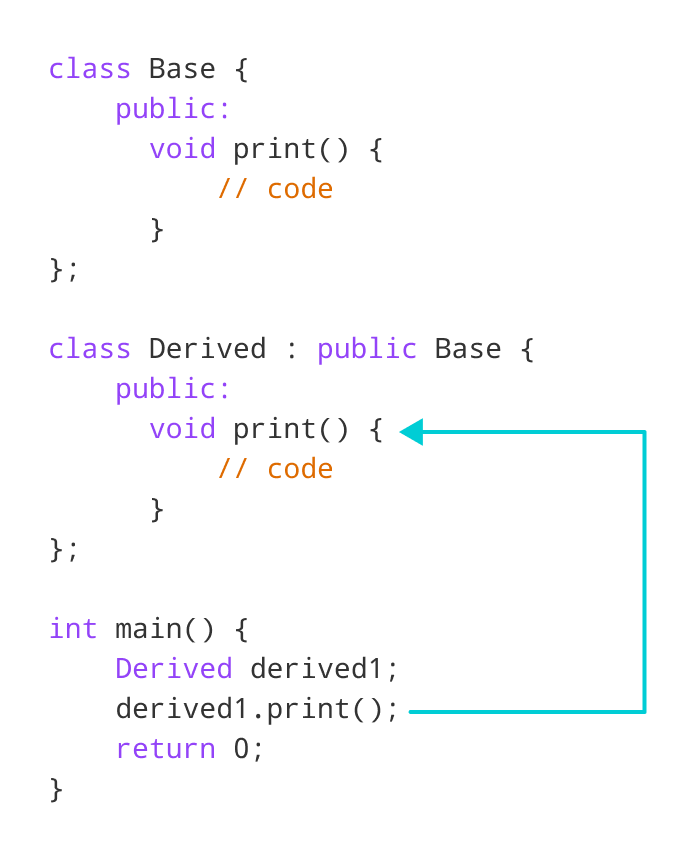
如我们所见,该函数被覆盖,因为我们从 Derived
的对象中调用了该函数 类。
如果我们调用 print()
Base
对象的函数 类,该函数不会被覆盖。
// Call function of Base class
Base base1;
base1.print(); // Output: Base Function
访问 C++ 中的覆盖函数
要访问基类的重写函数,我们使用范围解析运算符 ::
.
我们还可以通过使用基类的指针指向派生类的对象,然后从该指针调用函数来访问被覆盖的函数。
示例 2:对基类的 C++ 访问覆盖函数
// C++ program to access overridden function
// in main() using the scope resolution operator ::
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1, derived2;
derived1.print();
// access print() function of the Base class
derived2.Base::print();
return 0;
}
输出
Derived Function Base Function
这里,这个说法
derived2.Base::print();
访问 print()
Base 类的函数。
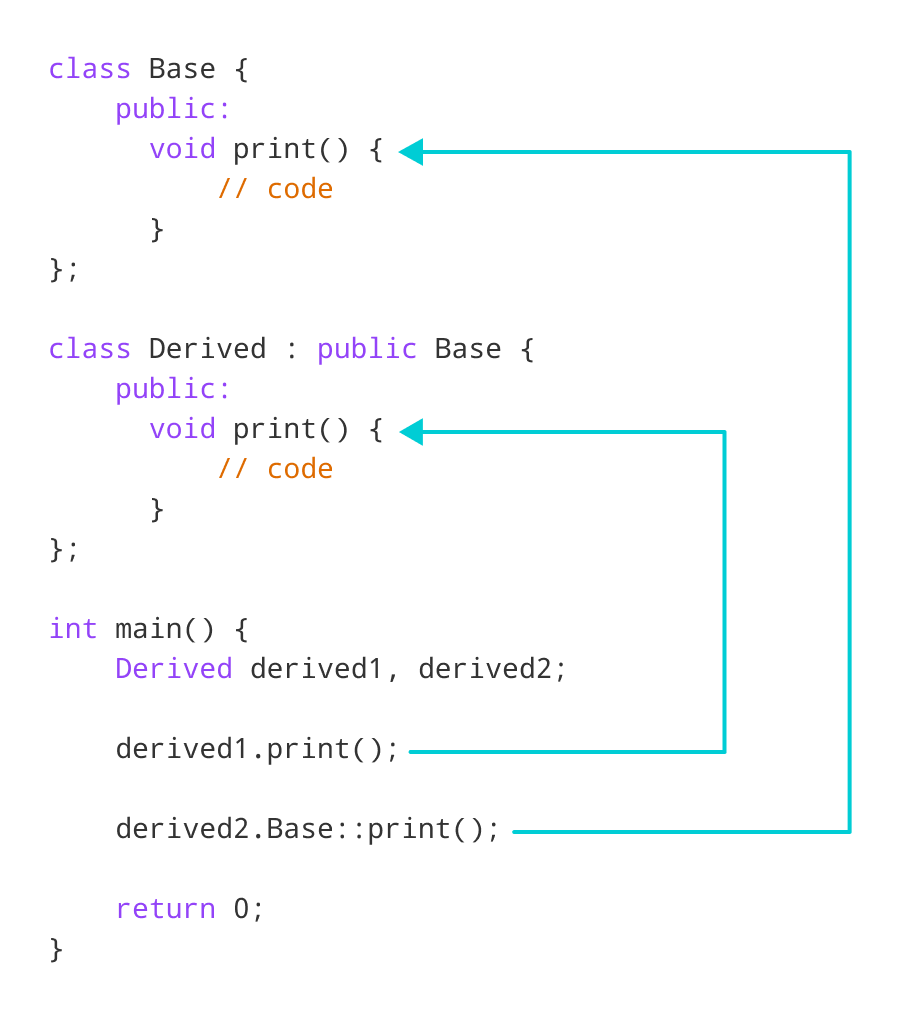
示例 3:C++ 从派生类调用重写函数
// C++ program to call the overridden function
// from a member function of the derived class
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
// call overridden function
Base::print();
}
};
int main() {
Derived derived1;
derived1.print();
return 0;
}
输出
Derived Function Base Function
在这个程序中,我们调用了 Derived
中的重写函数 类本身。
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
Base::print();
}
};
注意代码 Base::print();
,它调用 Derived
中的覆盖函数 类。
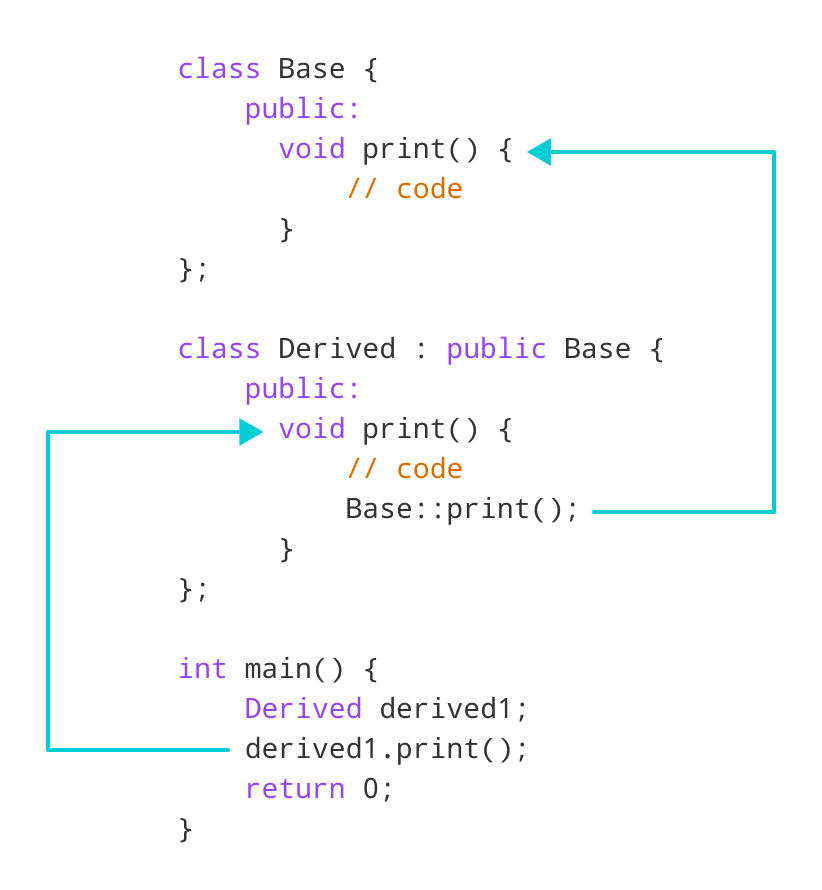
示例 4:C++ 使用指针调用重写函数
// C++ program to access overridden function using pointer
// of Base type that points to an object of Derived class
#include <iostream>
using namespace std;
class Base {
public:
void print() {
cout << "Base Function" << endl;
}
};
class Derived : public Base {
public:
void print() {
cout << "Derived Function" << endl;
}
};
int main() {
Derived derived1;
// pointer of Base type that points to derived1
Base* ptr = &derived1;
// call function of Base class using ptr
ptr->print();
return 0;
}
输出
Base Function
在这个程序中,我们创建了一个Base
的指针 名为 ptr 的类型 .这个指针指向 Derived
对象 derived1 .
// pointer of Base type that points to derived1
Base* ptr = &derived1;
当我们调用 print()
使用 ptr 的函数 ,它从 Base
调用被覆盖的函数 .
// call function of Base class using ptr
ptr->print();
这是因为即使 ptr 指向 Derived
对象,它实际上是 Base
类型。所以,它调用了Base
的成员函数 .
为了覆盖 Base
函数而不是访问它,我们需要在 Base
中使用虚函数 类。
C语言