Python for 循环
Python 循环
在本文中,您将学习使用 for 循环的不同变体来迭代一系列元素。
视频:Python for Loop
Python 中的 for 循环是什么?
Python 中的 for 循环用于迭代序列(列表、元组、字符串)或其他可迭代对象。遍历一个序列称为遍历。
for循环的语法
for val in sequence:
loop body
这里,val
是在每次迭代时取序列内项的值的变量。
循环继续,直到我们到达序列中的最后一项。 for 循环的主体与其余代码使用缩进分隔。
for循环流程图
<图>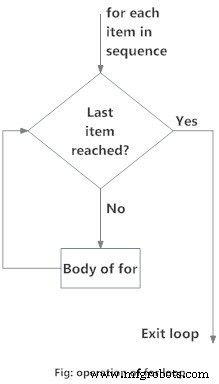
示例:Python for Loop
# Program to find the sum of all numbers stored in a list
# List of numbers
numbers = [6, 5, 3, 8, 4, 2, 5, 4, 11]
# variable to store the sum
sum = 0
# iterate over the list
for val in numbers:
sum = sum+val
print("The sum is", sum)
当你运行程序时,输出将是:
The sum is 48
range() 函数
我们可以使用 range()
生成一个数字序列 功能。 range(10)
将生成从 0 到 9 的数字(10 个数字)。
我们还可以将开始、停止和步长定义为 range(start, stop,step_size)
.如果未提供,step_size 默认为 1。
range
对象在某种意义上是“惰性的”,因为它不会在我们创建它时生成它“包含”的每个数字。但是,它不是迭代器,因为它支持 in
, len
和 __getitem__
操作。
此函数不会将所有值存储在内存中;这将是低效的。因此它会记住开始、停止、步长并在运行中生成下一个数字。
要强制此函数输出所有项目,我们可以使用函数 list()
.
下面的例子将阐明这一点。
print(range(10))
print(list(range(10)))
print(list(range(2, 8)))
print(list(range(2, 20, 3)))
输出
range(0, 10) [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] [2, 3, 4, 5, 6, 7] [2, 5, 8, 11, 14, 17]
我们可以使用 range()
for
中的函数 循环遍历一系列数字。它可以与 len()
结合使用 使用索引遍历序列的函数。这是一个例子。
# Program to iterate through a list using indexing
genre = ['pop', 'rock', 'jazz']
# iterate over the list using index
for i in range(len(genre)):
print("I like", genre[i])
输出
I like pop I like rock I like jazz
for 循环与 else
一个 for
循环可以有一个可选的 else
块为好。 else
如果 for 循环中使用的序列中的项目耗尽,则执行部分。
break 关键字可用于停止 for 循环。在这种情况下,else 部分将被忽略。
因此,如果没有发生中断,则 for 循环的 else 部分会运行。
这里有一个例子来说明这一点。
digits = [0, 1, 5]
for i in digits:
print(i)
else:
print("No items left.")
当你运行程序时,输出将是:
0 1 5 No items left.
在这里,for 循环打印列表中的项目,直到循环结束。当 for 循环耗尽时,它会执行 else
中的代码块 并打印
No items left.
这个for...else
语句可以与 break
一起使用 运行 else
的关键字 仅当 break
关键字未执行。举个例子吧:
# program to display student's marks from record
student_name = 'Soyuj'
marks = {'James': 90, 'Jules': 55, 'Arthur': 77}
for student in marks:
if student == student_name:
print(marks[student])
break
else:
print('No entry with that name found.')
输出
No entry with that name found.
Python