Python if...else 语句
Python if...else 语句
在本文中,您将学习使用不同形式的 if..else 语句在 Python 程序中创建决策。
视频:Python if...else 语句
Python 中的 if...else 语句是什么?
当我们想要仅在满足特定条件时执行代码时,就需要做出决策。
if…elif…else
语句在 Python 中用于决策。
Python if 语句语法
if test expression: statement(s)
在这里,程序评估 test expression
并且仅当测试表达式为 True
时才会执行语句 .
如果测试表达式是 False
,则不执行语句。
在 Python 中,if
的主体 语句由缩进表示。正文以缩进开头,第一条未缩进的行标记结束。
Python 将非零值解释为 True
. None
和 0
被解释为 False
.
Python if 语句流程图
<图>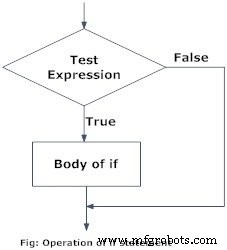
示例:Python if 语句
# If the number is positive, we print an appropriate message
num = 3
if num > 0:
print(num, "is a positive number.")
print("This is always printed.")
num = -1
if num > 0:
print(num, "is a positive number.")
print("This is also always printed.")
当你运行程序时,输出将是:
3 is a positive number This is always printed This is also always printed.
在上面的例子中,num > 0
是测试表达式。
if
的正文 仅当计算结果为 True
时才执行 .
当变量 num 等于 3,测试表达式为真,if
正文中的语句 被执行。
如果变量 num 等于 -1,测试表达式为假,if
正文中的语句 被跳过。
print()
语句在 if
之外 块(未缩进)。因此,无论测试表达式如何,它都会执行。
Python if...else 语句
if...else 的语法
if test expression: Body of if else: Body of else
if..else
语句计算 test expression
并将执行 if
的主体 仅当测试条件为 True
.
如果条件是False
, else
的主体 被执行。缩进用于分隔块。
Python if..else 流程图
<图>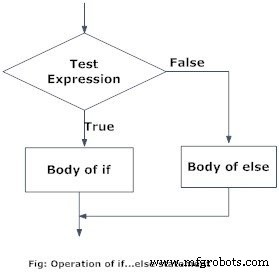
if...else 示例
# Program checks if the number is positive or negative
# And displays an appropriate message
num = 3
# Try these two variations as well.
# num = -5
# num = 0
if num >= 0:
print("Positive or Zero")
else:
print("Negative number")
输出
Positive or Zero
在上面的例子中,当 num 等于 3,测试表达式为真,if
的正文 被执行并且 body
of else 被跳过。
如果 num 等于-5,测试表达式为假,else
的正文 被执行,if
的主体 被跳过了。
如果 num 等于0,测试表达式为真,if
的正文 被执行并且 body
of else 被跳过。
Python if...elif...else 语句
if...elif...else 的语法
if test expression: Body of if elif test expression: Body of elif else: Body of else
elif
是 else if 的缩写。它允许我们检查多个表达式。
如果 if
的条件 是 False
,它检查下一个 elif
的条件 块等等。
如果所有条件都是False
, else 的主体被执行。
几个if...elif...else
中只有一个块 块根据条件执行。
if
块只能有一个 else
堵塞。但它可以有多个elif
块。
if...elif...else的流程图
<图>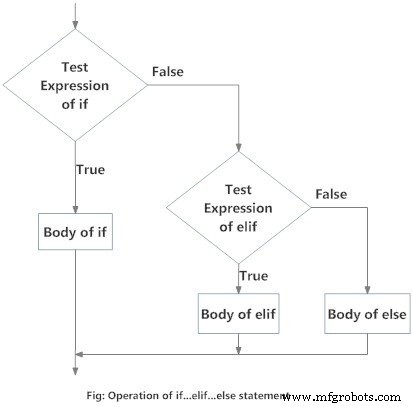
if...elif...else 示例
'''In this program,
we check if the number is positive or
negative or zero and
display an appropriate message'''
num = 3.4
# Try these two variations as well:
# num = 0
# num = -4.5
if num > 0:
print("Positive number")
elif num == 0:
print("Zero")
else:
print("Negative number")
当变量 num 是正数, 正数 被打印出来了。
如果 num 等于0, 零 被打印出来了。
如果 num 是负数, 负数 被打印出来了。
Python 嵌套 if 语句
我们可以有一个 if...elif...else
另一个 if...elif...else
中的语句 陈述。这在计算机编程中称为嵌套。
任意数量的这些语句都可以相互嵌套。缩进是确定嵌套级别的唯一方法。它们可能会让人感到困惑,因此除非必要,否则必须避免使用它们。
Python 嵌套 if 示例
'''In this program, we input a number
check if the number is positive or
negative or zero and display
an appropriate message
This time we use nested if statement'''
num = float(input("Enter a number: "))
if num >= 0:
if num == 0:
print("Zero")
else:
print("Positive number")
else:
print("Negative number")
输出 1
Enter a number: 5 Positive number
输出 2
Enter a number: -1 Negative number
输出 3
Enter a number: 0 Zero
Python