C# 中断语句
C# break 语句
在本教程中,您将通过示例了解 C# 中的 break 语句。
在 C# 中,我们使用 break 语句来终止循环。
众所周知,循环会遍历代码块,直到测试表达式为假。但是,有时我们可能需要在不检查测试表达式的情况下立即终止循环。
在这种情况下,使用 break 语句。 break语句的语法是,
break;
在我们了解break
之前 ,请务必了解
- for循环
- 如果...否则
- while 循环
示例:带有 for 循环的 C# break 语句
using System;
namespace CSharpBreak {
class Program {
static void Main(string[] args) {
for (int i = 1; i <= 4; ++i) {
// terminates the loop
if (i == 3) {
break;
}
Console.WriteLine(i);
}
Console.ReadLine();
}
}
}
输出
1 2
在上面的程序中,我们的 for
循环运行 4 i = 1
的次数 到4 .然而,当 i
等于 3 ,遇到break语句。
if (i == 3) {
break;
}
现在,循环突然终止。所以,我们只得到 1 和2 作为输出。
注意 :break 语句与 if..else 等决策语句一起使用。
示例:带有 while 循环的 C# break 语句
using System;
namespace WhileBreak {
class Program {
static void Main(string[] args) {
int i = 1;
while (i <= 5) {
Console.WriteLine(i);
i++;
if (i == 4) {
// terminates the loop
break;
}
}
Console.ReadLine();
}
}
}
输出
1 2 3
在上面的例子中,我们创建了一个 while
应该从 i = 1 to 5
运行的循环 .
但是,当 i
等于 4 , break
遇到语句。
if (i == 4) {
break;
}
现在,while 循环终止。
C#中break语句的工作
<图>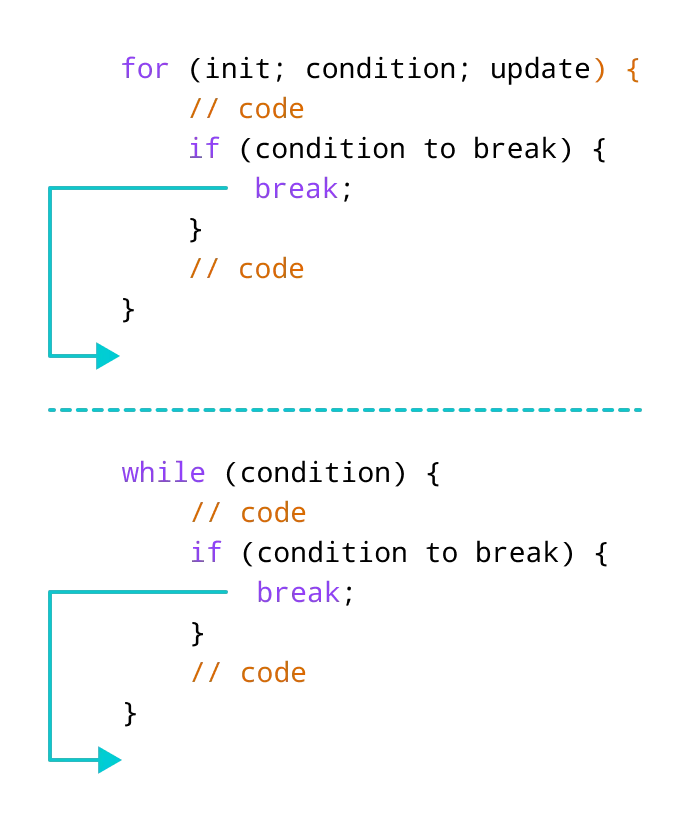
带有嵌套循环的break语句
我们也可以使用 break
带有嵌套循环的语句。例如,
using System;
namespace NestedBreak {
class Program {
static void Main(string[] args) {
int sum = 0;
for(int i = 1; i <= 3; i++) { //outer loop
// inner loop
for(int j = 1; j <= 3; j++) {
if (i == 2) {
break;
}
Console.WriteLine("i = " + i + " j = " +j);
}
}
Console.ReadLine();
}
}
}
输出
i = 1 j = 1 i = 1 j = 2 i = 1 j = 3 i = 3 j = 1 i = 3 j = 2 i = 3 j = 3
在上面的例子中,我们在 for
内部使用了 break 语句 环形。这里,break语句在i == 2
时执行 .
因此,i = 2
的值 从不打印。
注意 :break 语句只终止内部 for
环形。这是因为我们使用了 break
内循环中的语句。
如果您想了解嵌套循环的工作原理,请访问 C# Nested Loops。
用 foreach 循环中断
我们也可以使用 break
带有 foreach 循环的语句。例如,
using System;
namespace ForEachBreak {
class Program {
static void Main(string[] args) {
int[] num = { 1, 2, 3, 4, 5 };
// use of for each loop
foreach(int number in num) {
// terminates the loop
if(number==3) {
break;
}
Console.WriteLine(number);
}
}
}
}
输出
1 2
在上面的示例中,我们创建了一个包含值的数组:1 , 2 , 3 , 4 , 5 .在这里,我们使用了 foreach
循环打印数组的每个元素。
但是,循环只打印 1 和2 .这是因为当数字等于 3 ,break语句被执行。
if (number == 3) {
break;
}
这会立即终止 foreach 循环。
用 Switch 语句中断
我们也可以使用 break
switch case 语句中的语句。例如,
using System;
namespace ConsoleApp1 {
class Program {
static void Main(string[] args) {
char ch='e';
switch (ch) {
case 'a':
Console.WriteLine("Vowel");
break;
case 'e':
Console.WriteLine("Vowel");
break;
case 'i':
Console.WriteLine("Vowel");
break;
case 'o':
Console.WriteLine("Vowel");
break;
case 'u':
Console.WriteLine("Vowel");
break;
default:
Console.WriteLine("Not a vowel");
}
}
}
}
输出
Vowel
在这里,我们使用了 break
每个案例中的声明。它可以帮助我们在找到匹配的 case 时终止 switch 语句。
要了解更多信息,请访问 C# switch 语句。
C语言