C++ switch..case 语句
C++ switch..case 语句
在本教程中,我们将通过一些示例了解 switch 语句及其在 C++ 编程中的工作原理。
switch
语句允许我们在许多备选方案中执行一段代码。
switch
的语法 C++中的语句是:
switch (expression) {
case constant1:
// code to be executed if
// expression is equal to constant1;
break;
case constant2:
// code to be executed if
// expression is equal to constant2;
break;
.
.
.
default:
// code to be executed if
// expression doesn't match any constant
}
switch 语句是如何工作的?
expression
评估一次并与每个 case
的值进行比较 标签。
- 如果有匹配,则执行匹配标签后的对应代码。例如,如果变量的值等于
constant2
,case constant2:
之后的代码 一直执行,直到遇到 break 语句。 - 如果不匹配,则
default:
之后的代码 被执行。
注意 :我们可以用 if...else..if
做同样的事情 梯子。但是,switch
的语法 语句更简洁,更易于阅读和编写。
switch语句流程图
<图>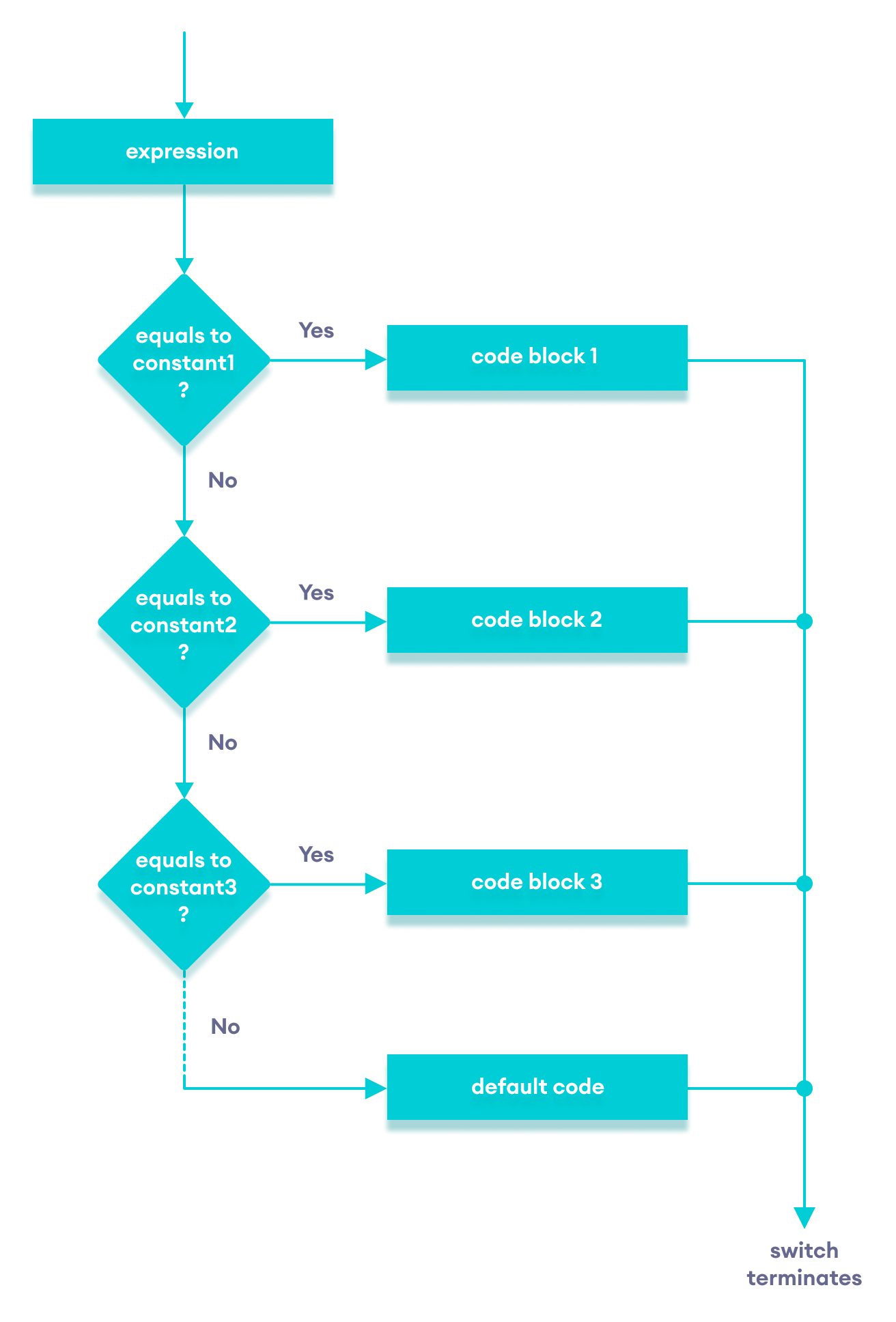
示例:使用switch语句创建计算器
// Program to build a simple calculator using switch Statement
#include <iostream>
using namespace std;
int main() {
char oper;
float num1, num2;
cout << "Enter an operator (+, -, *, /): ";
cin >> oper;
cout << "Enter two numbers: " << endl;
cin >> num1 >> num2;
switch (oper) {
case '+':
cout << num1 << " + " << num2 << " = " << num1 + num2;
break;
case '-':
cout << num1 << " - " << num2 << " = " << num1 - num2;
break;
case '*':
cout << num1 << " * " << num2 << " = " << num1 * num2;
break;
case '/':
cout << num1 << " / " << num2 << " = " << num1 / num2;
break;
default:
// operator is doesn't match any case constant (+, -, *, /)
cout << "Error! The operator is not correct";
break;
}
return 0;
}
输出 1
Enter an operator (+, -, *, /): + Enter two numbers: 2.3 4.5 2.3 + 4.5 = 6.8
输出 2
Enter an operator (+, -, *, /): - Enter two numbers: 2.3 4.5 2.3 - 4.5 = -2.2
输出 3
Enter an operator (+, -, *, /): * Enter two numbers: 2.3 4.5 2.3 * 4.5 = 10.35
输出 4
Enter an operator (+, -, *, /): / Enter two numbers: 2.3 4.5 2.3 / 4.5 = 0.511111
输出 5
Enter an operator (+, -, *, /): ? Enter two numbers: 2.3 4.5 Error! The operator is not correct.
在上面的程序中,我们使用的是 switch...case
执行加减乘除的语句。
这个程序如何运作
- 我们首先提示用户输入所需的运算符。然后将此输入存储在
char
名为 oper 的变量 . - 然后我们提示用户输入两个数字,它们存储在浮点变量num1中 和 num2 .
switch
然后使用语句检查用户输入的运算符:- 如果用户输入
+
, 对数字执行加法。 - 如果用户输入
-
, 对数字执行减法。 - 如果用户输入
*
, 对数字进行乘法运算。 - 如果用户输入
/
, 对数字进行除法。 - 如果用户输入任何其他字符,则打印默认代码。
- 如果用户输入
注意 break
语句在每个 case
中使用 堵塞。这将终止 switch
声明。
如果 break
不使用语句,正确的case
之后的所有情况 被执行。
C语言