如何从 C++ 函数传递和返回对象?
如何从 C++ 函数中传递和返回对象?
在本教程中,我们将学习在 C++ 编程中将对象传递给函数并从函数返回对象。
在 C++ 编程中,我们可以像传递常规参数一样将对象传递给函数。
示例 1:C++ 将对象传递给函数
// C++ program to calculate the average marks of two students
#include <iostream>
using namespace std;
class Student {
public:
double marks;
// constructor to initialize marks
Student(double m) {
marks = m;
}
};
// function that has objects as parameters
void calculateAverage(Student s1, Student s2) {
// calculate the average of marks of s1 and s2
double average = (s1.marks + s2.marks) / 2;
cout << "Average Marks = " << average << endl;
}
int main() {
Student student1(88.0), student2(56.0);
// pass the objects as arguments
calculateAverage(student1, student2);
return 0;
}
输出
Average Marks = 72
在这里,我们通过了两个Student
对象 student1 和 student2 作为 calculateAverage()
的参数 功能。
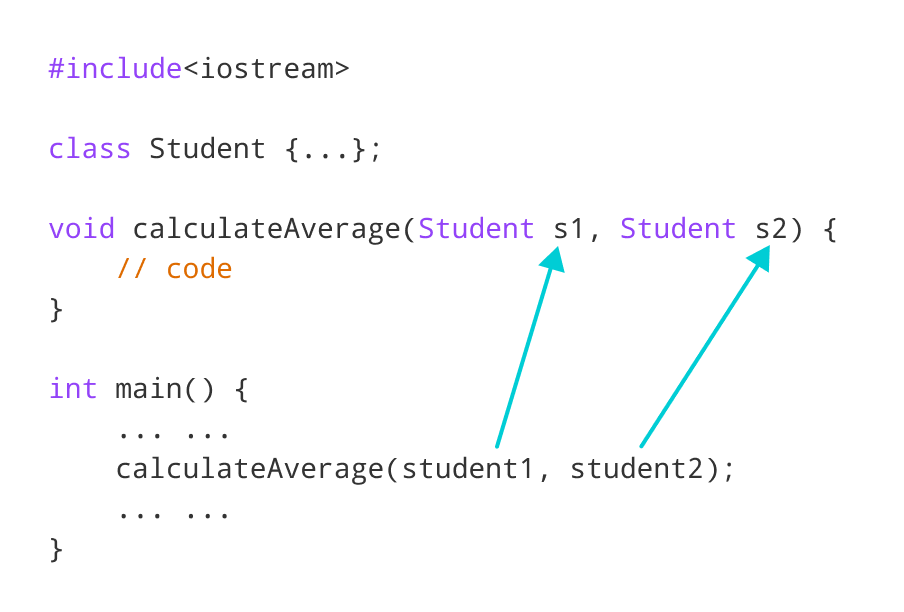
示例 2:C++ 从函数返回对象
#include <iostream>
using namespace std;
class Student {
public:
double marks1, marks2;
};
// function that returns object of Student
Student createStudent() {
Student student;
// Initialize member variables of Student
student.marks1 = 96.5;
student.marks2 = 75.0;
// print member variables of Student
cout << "Marks 1 = " << student.marks1 << endl;
cout << "Marks 2 = " << student.marks2 << endl;
return student;
}
int main() {
Student student1;
// Call function
student1 = createStudent();
return 0;
}
输出
Marks1 = 96.5 Marks2 = 75<图>
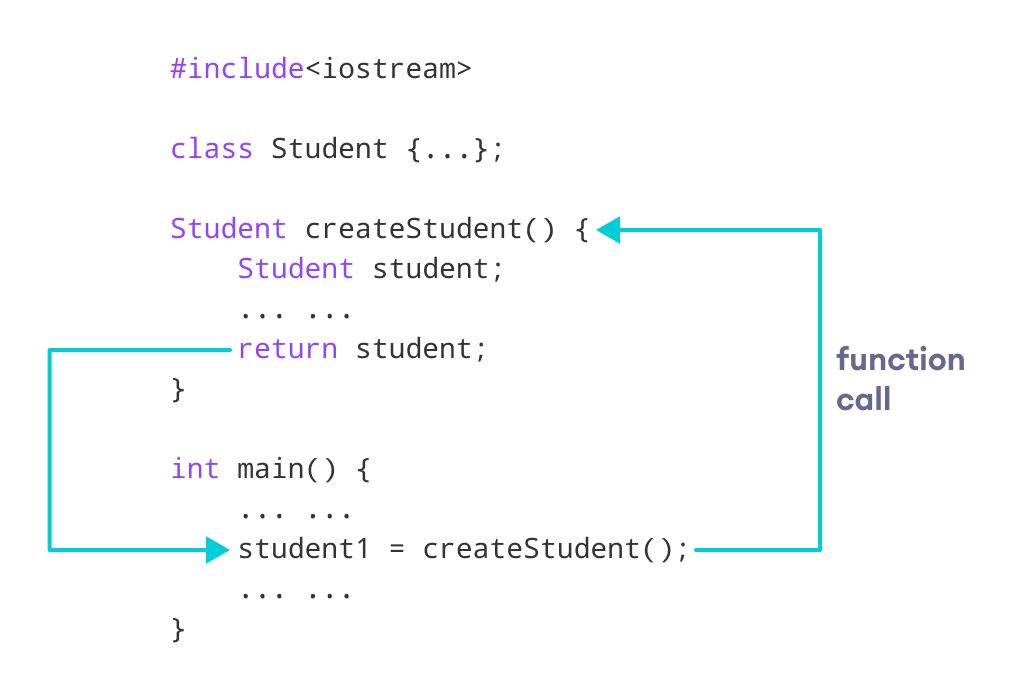
在这个程序中,我们创建了一个函数createStudent()
返回 Student
的对象 类。
我们调用了 createStudent()
来自 main()
方法。
// Call function
student1 = createStudent();
在这里,我们存储 createStudent()
返回的对象 student1 中的方法 .
C语言