C++ 运算符重载
C++ 运算符重载
在本教程中,我们将通过示例了解运算符重载。
在 C++ 中,我们可以改变运算符为用户定义的类型(如对象和结构)工作的方式。这称为运算符重载 .例如,
假设我们创建了三个对象 c1 , c2 和 结果 来自一个名为 Complex
的类 表示复数。
由于运算符重载允许我们改变运算符的工作方式,我们可以重新定义 +
运算符工作并使用它来添加 c1 的复数 和 c2 通过编写以下代码:
result = c1 + c2;
而不是像
result = c1.addNumbers(c2);
这使我们的代码直观易懂。
注意: 我们不能对像 int
这样的基本数据类型使用运算符重载 , float
, char
等等。
C++ 运算符重载的语法
为了重载一个操作符,我们使用一个特殊的 operator
功能。我们在类或结构中定义函数,我们希望重载运算符使用其对象/变量。
class className {
... .. ...
public
returnType operator symbol (arguments) {
... .. ...
}
... .. ...
};
在这里,
returnType
是函数的返回类型。operator
是一个关键字。symbol
是我们要重载的运算符。喜欢:+
,<
,-
,++
等。arguments
是传递给函数的参数。
一元运算符中的运算符重载
一元运算符仅对一个操作数进行操作。增量运算符 ++
和递减运算符 --
是一元运算符的例子。
示例1:++运算符(一元运算符)重载
// Overload ++ when used as prefix
#include <iostream>
using namespace std;
class Count {
private:
int value;
public:
// Constructor to initialize count to 5
Count() : value(5) {}
// Overload ++ when used as prefix
void operator ++ () {
++value;
}
void display() {
cout << "Count: " << value << endl;
}
};
int main() {
Count count1;
// Call the "void operator ++ ()" function
++count1;
count1.display();
return 0;
}
输出
Count: 6
在这里,当我们使用 ++count1;
, void operator ++ ()
叫做。这增加了 值 对象 count1 的属性 1.
注意: 当我们重载运算符时,我们可以使用它以任何我们喜欢的方式工作。例如,我们可以使用 ++
增加值 100。
然而,这使我们的代码混乱且难以理解。作为程序员,我们的工作是正确、一致且直观地使用运算符重载。
上述示例仅在 ++
时有效 用作前缀。制作 ++
作为后缀工作,我们使用这种语法。
void operator ++ (int) {
// code
}
注意 int
括号内。这是使用一元运算符作为后缀的语法;不是函数参数。
示例2:++运算符(一元运算符)重载
// Overload ++ when used as prefix and postfix
#include <iostream>
using namespace std;
class Count {
private:
int value;
public:
// Constructor to initialize count to 5
Count() : value(5) {}
// Overload ++ when used as prefix
void operator ++ () {
++value;
}
// Overload ++ when used as postfix
void operator ++ (int) {
value++;
}
void display() {
cout << "Count: " << value << endl;
}
};
int main() {
Count count1;
// Call the "void operator ++ (int)" function
count1++;
count1.display();
// Call the "void operator ++ ()" function
++count1;
count1.display();
return 0;
}
输出
Count: 6 Count: 7
示例 2 ++
时工作 用作前缀和后缀。但是,如果我们尝试做这样的事情是行不通的:
Count count1, result;
// Error
result = ++count1;
这是因为我们的算子函数的返回类型是void
.我们可以通过制作Count
来解决这个问题 作为运算符函数的返回类型。
// return Count when ++ used as prefix
Count operator ++ () {
// code
}
// return Count when ++ used as postfix
Count operator ++ (int) {
// code
}
示例3:运算符函数(++运算符)的返回值
#include <iostream>
using namespace std;
class Count {
private:
int value;
public
:
// Constructor to initialize count to 5
Count() : value(5) {}
// Overload ++ when used as prefix
Count operator ++ () {
Count temp;
// Here, value is the value attribute of the calling object
temp.value = ++value;
return temp;
}
// Overload ++ when used as postfix
Count operator ++ (int) {
Count temp;
// Here, value is the value attribute of the calling object
temp.value = value++;
return temp;
}
void display() {
cout << "Count: " << value << endl;
}
};
int main() {
Count count1, result;
// Call the "Count operator ++ ()" function
result = ++count1;
result.display();
// Call the "Count operator ++ (int)" function
result = count1++;
result.display();
return 0;
}
输出
Count: 6 Count: 6
在这里,我们使用以下代码进行前缀运算符重载:
// Overload ++ when used as prefix
Count operator ++ () {
Count temp;
// Here, value is the value attribute of the calling object
temp.value = ++value;
return temp;
}
后缀运算符重载的代码也类似。请注意,我们创建了一个对象 temp 并将其值返回给操作符函数。
另外,注意代码
temp.value = ++value;
变量值 属于 count1 main()
中的对象 因为 count1 正在调用函数,而 temp.value 属于 temp 对象。
二元运算符中的运算符重载
二元运算符对两个操作数起作用。例如,
result = num + 9;
这里,+
是对操作数 num 起作用的二元运算符 和 9
.
当我们使用代码重载用户定义类型的二元运算符时:
obj3 = obj1 + obj2;
使用 obj1 调用操作符函数 对象和 obj2 作为参数传递给函数。
示例 4:C++ 二元运算符重载
// C++ program to overload the binary operator +
// This program adds two complex numbers
#include <iostream>
using namespace std;
class Complex {
private:
float real;
float imag;
public:
// Constructor to initialize real and imag to 0
Complex() : real(0), imag(0) {}
void input() {
cout << "Enter real and imaginary parts respectively: ";
cin >> real;
cin >> imag;
}
// Overload the + operator
Complex operator + (const Complex& obj) {
Complex temp;
temp.real = real + obj.real;
temp.imag = imag + obj.imag;
return temp;
}
void output() {
if (imag < 0)
cout << "Output Complex number: " << real << imag << "i";
else
cout << "Output Complex number: " << real << "+" << imag << "i";
}
};
int main() {
Complex complex1, complex2, result;
cout << "Enter first complex number:\n";
complex1.input();
cout << "Enter second complex number:\n";
complex2.input();
// complex1 calls the operator function
// complex2 is passed as an argument to the function
result = complex1 + complex2;
result.output();
return 0;
}
输出
Enter first complex number: Enter real and imaginary parts respectively: 9 5 Enter second complex number: Enter real and imaginary parts respectively: 7 6 Output Complex number: 16+11i
在这个程序中,算子函数是:
Complex operator + (const Complex& obj) {
// code
}
取而代之的是,我们也可以编写如下函数:
Complex operator + (Complex obj) {
// code
}
然而,
- 使用
&
通过引用 complex2 使我们的代码高效 对象而不是在操作符函数中创建一个重复的对象。 - 使用
const
被认为是一种很好的做法,因为它可以防止操作员函数修改 complex2 .
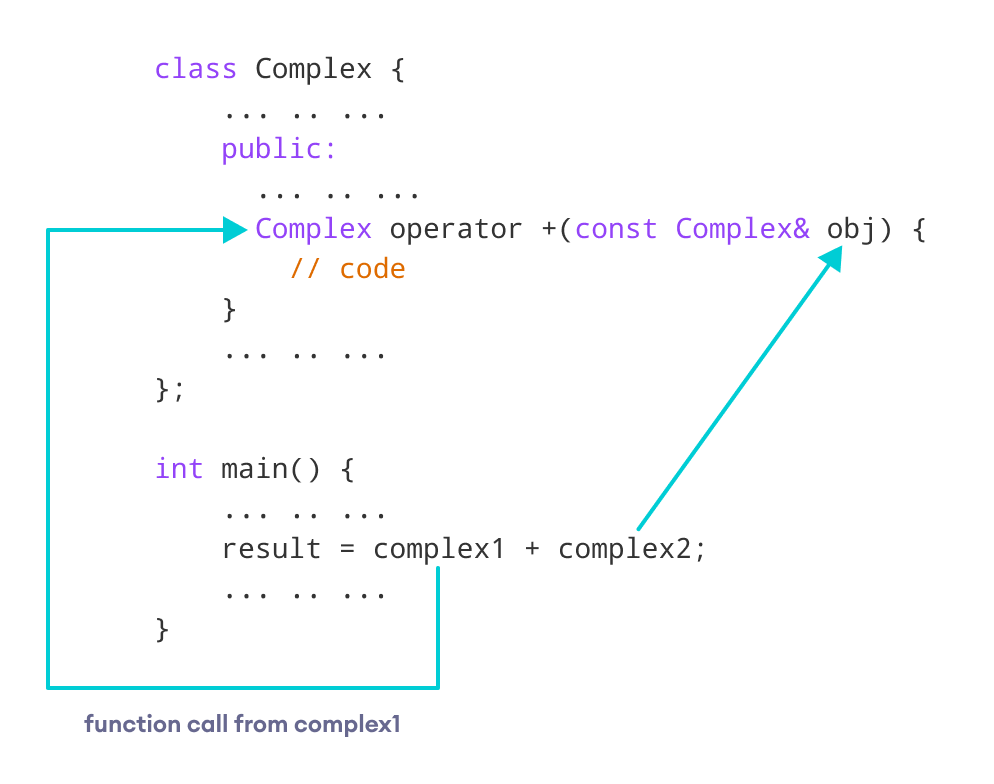
在 C++ 运算符重载中要记住的事情
- 两个运算符
=
和&
在 C++ 中已经默认重载。比如复制同一个类的对象,我们可以直接使用=
操作员。我们不需要创建运算符函数。 - 运算符重载不能改变运算符的优先级和结合性。但是,如果我们想更改评估顺序,则应使用括号。
- 有 4 个运算符不能在 C++ 中重载。他们是:
::
(范围分辨率).
(成员选择).*
(通过函数指针选择成员)?:
(三元运算符)
访问这些页面以了解更多信息:
- 如何正确重载增量运算符?
- 如何重载二元运算符 - 减去复数?
C语言