Verilog 语法
Verilog 中的词汇约定类似于 C,因为它包含一个标记流。词法标记可以由一个或多个字符组成,标记可以是注释、关键字、数字、字符串或空格。所有行都应以分号 ;
结尾 .
Verilog 区分大小写 ,所以 var_a 和 var_A 是不同的。
评论
在 Verilog 中有两种写注释的方法。
- 单行 注释以
//
开头 并告诉 Verilog 编译器将此点之后到行尾的所有内容都视为注释。 - 一个多行 注释以
/*
开头 并以*/
结尾 并且不能嵌套。
但是,单行注释可以嵌套在多行注释中。
// This is a single line comment
integer a; // Creates an int variable called a, and treats everything to the right of // as a comment
/*
This is a
multiple-line or
block comment
*/
/* This is /*
an invalid nested
block comment */
*/
/* However,
// this one is okay
*/
// This is also okay
///////////// Still okay
空格
空白是用于表示空格、制表符、换行符和换页符的字符的术语,通常被 Verilog 忽略,除非它分隔标记。事实上,这有助于代码的缩进,使其更易于阅读。
module dut; // 'module' is a keyword, // 'dut' is an identifier reg [8*6:1] name = "Hello!"; // The 2 spaces in the beginning are ignored
但是,字符串中不会忽略空格(空格)和制表符(来自 TAB 键)。在下面的示例中,字符串 由于字符串中保留了空格,名为 addr 的变量获得了值“Earth”。
// There is no space in the beginning of this line, // but there's a space in the string reg [8*6:1] addr = "Earth "; endmodule
运营商
共有三种类型的运算符:一元 , 二进制 , 和 三元或条件 .
- 一元运算符应出现在其操作数的左侧
- 二元运算符应出现在其操作数之间
- 条件运算符有两个独立的运算符来分隔三个操作数
x = ~y; // ~ is a unary operator, and y is the operand
x = y | z; // | is a binary operator, where y and z are its operands
x = (y > 5) ? w : z; // ?: is a ternary operator, and the expression (y>5), w and z are its operands
如果表达式 (y> 5) 为真,则变量 x 将获得 w 中的值 , 否则 z 中的值 .
数字格式
我们最熟悉用小数表示的数字。但是,数字也可以用 binary 表示 , 八进制 和十六进制 .默认情况下,Verilog 模拟器将数字视为小数。为了用不同的 radix 来表示它们 ,必须遵守一定的规则。
16 // Number 16 in decimal 0x10 // Number 16 in hexadecimal 10000 // Number 16 in binary 20 // Number 16 in octal
大小
大小数字如下所示,其中 size 仅以十进制写入以指定数字中的位数。
[size]'[base_format][number]
- base_format 可以是十进制('d 或 'D)、十六进制('h 或 'H)和八进制('o 或 'O),并指定 number 的基数 部分代表。
- 号码 指定为从 0、1、2 ... 9 的连续数字(十进制基本格式)和 0、1、2 .. 9、A、B、C、D、E、F (十六进制)。
3'b010; // size is 3, base format is binary ('b), and the number is 010 (indicates value 2 in binary) 3'd2; // size is 3, base format is decimal ('d) and the number is 2 (specified in decimals) 8'h70; // size is 8, base format is hexadecimal ('h) and the number is 0x70 (in hex) to represent decimal 112 9'h1FA; // size is 9, base format is hexadecimal ('h) and the number is 0x1FA (in hex) to represent decimal 506 4'hA = 4'd10 = 4'b1010 = 4'o12 // Decimal 10 can be represented in any of the four formats 8'd234 = 8'D234 // Legal to use either lower case or upper case for base format 32'hFACE_47B2; // Underscore (_) can be used to separate 16 bit numbers for readability
当基本格式为十六进制时,大写字母对于数字规范是合法的。
16'hcafe; // lowercase letters Valid 16'hCAFE; // uppercase letters Valid 32'h1D40_CAFE; // underscore can be used as separator between 4 letters Valid
无尺寸
没有 base_format 的数字 默认规范是十进制数 .没有 size 的数字 规范有一个默认位数,具体取决于模拟器和机器的类型。
integer a = 5423; // base format is not specified, a gets a decimal value of 5423
integer a = 'h1AD7; // size is not specified, because a is int (32 bits) value stored in a = 32'h0000_1AD7
否定
通过放置一个减号 -
来指定负数 在数字大小之前签名。 base_format 之间有减号是非法的 和号码 .
-6'd3; // 8-bit negative number stored as two's complement of 3 -6'sd9; // For signed maths 8'd-4; // Illegal
字符串
用双引号 " "
括起来的字符序列 称为字符串。不能拆分成多行,字符串中的每个字符占用1个字节存储。
"Hello World!" // String with 12 characters -> require 12 bytes "x + z" // String with 5 characters "How are you feeling today ?" // Illegal for a string to be split into multiple lines
标识符
标识符 是变量的名称,以便以后可以引用它们。它们由字母数字字符 [a-z][A-Z][0-9]
组成 , 下划线 _
或美元符号 $
并且区分大小写。它们不能以数字或美元符号开头。
integer var_a; // Identifier contains alphabets and underscore -> Valid integer $var_a; // Identifier starts with $ -> Invalid integer v$ar_a; // Identifier contains alphabets and $ -> Valid integer 2var; // Identifier starts with a digit -> Invalid integer var23_g; // Identifier contains alphanumeric characters and underscore -> Valid integer 23; // Identifier contains only numbers -> Invalid
关键词
关键字是为定义语言结构而保留的特殊标识符,并且是小写的。下面列出了重要的关键字。
<无脚本>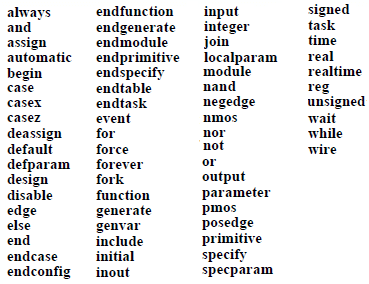
Verilog 修订
Verilog 多年来经历了一些修改,从 1995 年到 2001 年进行了更多的添加,如下所示。
<无脚本>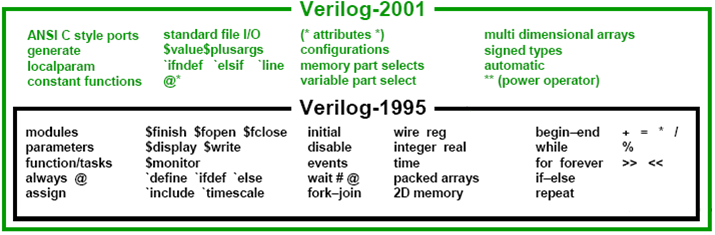
Verilog